mirror of
https://github.com/discourse/discourse.git
synced 2025-05-29 01:31:35 +08:00
DEV: Support validations options for string and numeral types (#25719)
Why this change? This commit updates `ThemeSettingsObjectValidator` to validate a property's value against the validations listed in the schema. For string types, `min_length`, `max_length` and `url` are supported. For integer and float types, `min` and `max` are supported.
This commit is contained in:
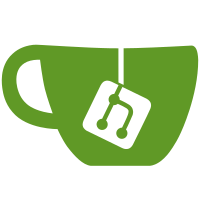
committed by
GitHub
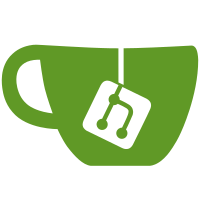
parent
3894ee6cb6
commit
bf3c4b634a
@ -139,6 +139,29 @@ RSpec.describe ThemeSettingsObjectValidator do
|
||||
described_class.new(schema: schema, object: { float_property: "string" }).validate,
|
||||
).to eq(float_property: ["must be a float"])
|
||||
end
|
||||
|
||||
it "should return the right hash of error messages when integer property does not satisfy min or max validations" do
|
||||
schema = {
|
||||
name: "section",
|
||||
properties: {
|
||||
float_property: {
|
||||
type: "float",
|
||||
validations: {
|
||||
min: 5.5,
|
||||
max: 11.5,
|
||||
},
|
||||
},
|
||||
},
|
||||
}
|
||||
|
||||
expect(described_class.new(schema: schema, object: { float_property: 4.5 }).validate).to eq(
|
||||
float_property: ["must be larger than or equal to 5.5"],
|
||||
)
|
||||
|
||||
expect(
|
||||
described_class.new(schema: schema, object: { float_property: 12.5 }).validate,
|
||||
).to eq(float_property: ["must be smaller than or equal to 11.5"])
|
||||
end
|
||||
end
|
||||
|
||||
context "for integer properties" do
|
||||
@ -159,6 +182,48 @@ RSpec.describe ThemeSettingsObjectValidator do
|
||||
described_class.new(schema: schema, object: { integer_property: 1.0 }).validate,
|
||||
).to eq(integer_property: ["must be an integer"])
|
||||
end
|
||||
|
||||
it "should not return any error messages when the value of the integer property satisfies min and max validations" do
|
||||
schema = {
|
||||
name: "section",
|
||||
properties: {
|
||||
integer_property: {
|
||||
type: "integer",
|
||||
validations: {
|
||||
min: 5,
|
||||
max: 10,
|
||||
},
|
||||
},
|
||||
},
|
||||
}
|
||||
|
||||
expect(described_class.new(schema: schema, object: { integer_property: 6 }).validate).to eq(
|
||||
{},
|
||||
)
|
||||
end
|
||||
|
||||
it "should return the right hash of error messages when integer property does not satisfy min or max validations" do
|
||||
schema = {
|
||||
name: "section",
|
||||
properties: {
|
||||
integer_property: {
|
||||
type: "integer",
|
||||
validations: {
|
||||
min: 5,
|
||||
max: 10,
|
||||
},
|
||||
},
|
||||
},
|
||||
}
|
||||
|
||||
expect(described_class.new(schema: schema, object: { integer_property: 4 }).validate).to eq(
|
||||
integer_property: ["must be larger than or equal to 5"],
|
||||
)
|
||||
|
||||
expect(
|
||||
described_class.new(schema: schema, object: { integer_property: 11 }).validate,
|
||||
).to eq(integer_property: ["must be smaller than or equal to 10"])
|
||||
end
|
||||
end
|
||||
|
||||
context "for string properties" do
|
||||
@ -171,10 +236,72 @@ RSpec.describe ThemeSettingsObjectValidator do
|
||||
end
|
||||
|
||||
it "should return the right hash of error messages when value of property is not of type string" do
|
||||
schema = { name: "section", properties: { string_property: { type: "string" } } }
|
||||
|
||||
expect(described_class.new(schema: schema, object: { string_property: 1 }).validate).to eq(
|
||||
string_property: ["must be a string"],
|
||||
)
|
||||
end
|
||||
|
||||
it "should return the right hash of error messages when string property does not statisfy url validation" do
|
||||
schema = {
|
||||
name: "section",
|
||||
properties: {
|
||||
string_property: {
|
||||
type: "string",
|
||||
validations: {
|
||||
url: true,
|
||||
},
|
||||
},
|
||||
},
|
||||
}
|
||||
|
||||
expect(
|
||||
described_class.new(schema: schema, object: { string_property: "not a url" }).validate,
|
||||
).to eq(string_property: ["must be a valid URL"])
|
||||
end
|
||||
|
||||
it "should not return any error messages when the value of the string property satisfies min_length and max_length validations" do
|
||||
schema = {
|
||||
name: "section",
|
||||
properties: {
|
||||
string_property: {
|
||||
type: "string",
|
||||
validations: {
|
||||
min_length: 5,
|
||||
max_length: 10,
|
||||
},
|
||||
},
|
||||
},
|
||||
}
|
||||
|
||||
expect(
|
||||
described_class.new(schema: schema, object: { string_property: "123456" }).validate,
|
||||
).to eq({})
|
||||
end
|
||||
|
||||
it "should return the right hash of error messages when string property does not satisfy min_length or max_length validations" do
|
||||
schema = {
|
||||
name: "section",
|
||||
properties: {
|
||||
string_property: {
|
||||
type: "string",
|
||||
validations: {
|
||||
min_length: 5,
|
||||
max_length: 10,
|
||||
},
|
||||
},
|
||||
},
|
||||
}
|
||||
|
||||
expect(
|
||||
described_class.new(schema: schema, object: { string_property: "1234" }).validate,
|
||||
).to eq(string_property: ["must be at least 5 characters long"])
|
||||
|
||||
expect(
|
||||
described_class.new(schema: schema, object: { string_property: "12345678910" }).validate,
|
||||
).to eq(string_property: ["must be at most 10 characters long"])
|
||||
end
|
||||
end
|
||||
end
|
||||
end
|
||||
|
Reference in New Issue
Block a user