mirror of
https://github.com/discourse/discourse.git
synced 2025-07-14 01:10:53 +08:00
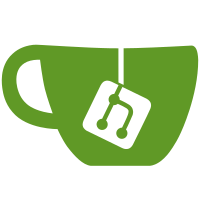
This commit adds - `topic_localization` containing its topic, a locale, title, and fancy_title - `post_localization` containing its post, a locale, raw, cooked, the associated post's version - and also APIs to add them Reviewer note: We may ask ourselves "why create separate models instead of one that is generic?" but the different localizable models may be vastly different. For example in posts we only have raw that we need to translate, and topics we have only title, but for categories we have name and description. Then, we may ask ourselves "why not create a polymorphic one that takes in model and column name?" and then we end up with the same thing as what we have currently which is custom fields (which is a mess in itself). Also, when replacing the untranslated content to the translated one, we may find it easier to just `join` + `coalesce` on the dedicated table - it would be a much simpler query than polymorphism.
44 lines
1.2 KiB
Ruby
44 lines
1.2 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
describe PostLocalizationUpdater do
|
|
fab!(:user)
|
|
fab!(:post) { Fabricate(:post, version: 99) }
|
|
fab!(:group)
|
|
fab!(:post_localization) do
|
|
Fabricate(:post_localization, post: post, locale: "ja", raw: "古いバージョン")
|
|
end
|
|
|
|
let(:locale) { "ja" }
|
|
let(:new_raw) { "新しいバージョンです" }
|
|
|
|
before do
|
|
SiteSetting.experimental_content_localization = true
|
|
SiteSetting.experimental_content_localization_allowed_groups = group.id.to_s
|
|
group.add(user)
|
|
end
|
|
|
|
it "updates an existing localization" do
|
|
localization =
|
|
described_class.update(post_id: post.id, locale: locale, raw: new_raw, user: user)
|
|
|
|
expect(localization).to have_attributes(
|
|
raw: new_raw,
|
|
cooked: PrettyText.cook(new_raw),
|
|
localizer_user_id: user.id,
|
|
post_version: post.version,
|
|
)
|
|
end
|
|
|
|
it "raises not found if the localization is missing" do
|
|
expect {
|
|
described_class.update(post_id: post.id, locale: "nope", raw: new_raw, user: user)
|
|
}.to raise_error(Discourse::NotFound)
|
|
end
|
|
|
|
it "raises not found if the post is missing" do
|
|
expect {
|
|
described_class.update(post_id: -1, locale: locale, raw: new_raw, user: user)
|
|
}.to raise_error(Discourse::NotFound)
|
|
end
|
|
end
|