mirror of
https://github.com/flarum/framework.git
synced 2025-05-28 19:06:35 +08:00
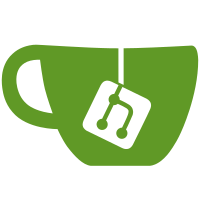
New stuff: - Signup + email confirmation. - Updated authentication strategy with remember cookies. closes #5 - New search system with some example gambits! This is cool - check out the source. Fulltext drivers will be implemented as decorators overriding the EloquentPostRepository’s findByContent method. - Lay down the foundation for bootstrapping the Ember app. - Update Web layer’s asset manager to properly publish CSS/JS files. - Console commands to run installation migrations and seeds. Refactoring: - New structure: move models, repositories, commands, and events into their own namespaces, rather than grouping by entity. - All events are classes. - Use L5 middleware and command bus implementations. - Clearer use of repositories and the Active Record pattern. Repositories are used only for retrieval of ActiveRecord objects, and then save/delete operations are called directly on those ActiveRecords. This way, we don’t over-abstract at the cost of Eloquent magic, but testing is still easy. - Refactor of Web layer so that it uses the Actions routing architecture. - “Actor” concept instead of depending on Laravel’s Auth. - General cleanup!
63 lines
1.7 KiB
PHP
63 lines
1.7 KiB
PHP
<?php namespace Flarum\Api\Actions\Posts;
|
|
|
|
use Illuminate\Database\Eloquent\ModelNotFoundException;
|
|
use Flarum\Core\Repositories\PostRepositoryInterface;
|
|
use Flarum\Core\Support\Actor;
|
|
use Flarum\Api\Actions\BaseAction;
|
|
use Flarum\Api\Actions\ApiParams;
|
|
use Flarum\Api\Serializers\PostSerializer;
|
|
|
|
class IndexAction extends BaseAction
|
|
{
|
|
use GetsPostsForDiscussion;
|
|
|
|
/**
|
|
* The post repository.
|
|
*
|
|
* @var Post
|
|
*/
|
|
protected $posts;
|
|
|
|
/**
|
|
* Instantiate the action.
|
|
*
|
|
* @param Post $posts
|
|
*/
|
|
public function __construct(Actor $actor, PostRepositoryInterface $posts)
|
|
{
|
|
$this->actor = $actor;
|
|
$this->posts = $posts;
|
|
}
|
|
|
|
/**
|
|
* Show posts from a discussion, or by providing an array of IDs.
|
|
*
|
|
* @return Response
|
|
*/
|
|
protected function run(ApiParams $params)
|
|
{
|
|
$postIds = (array) $params->get('ids');
|
|
$include = ['user', 'user.groups', 'editUser', 'hideUser'];
|
|
$user = $this->actor->getUser();
|
|
|
|
if (count($postIds)) {
|
|
$posts = $this->posts->findByIds($postIds, $user);
|
|
} else {
|
|
$discussionId = $params->get('discussions');
|
|
$posts = $this->getPostsForDiscussion($params, $discussionId, $user);
|
|
}
|
|
|
|
if (! count($posts)) {
|
|
throw new ModelNotFoundException;
|
|
}
|
|
|
|
// Finally, we can set up the post serializer and use it to create
|
|
// a post resource or collection, depending on how many posts were
|
|
// requested.
|
|
$serializer = new PostSerializer($include);
|
|
$document = $this->document()->setPrimaryElement($serializer->collection($posts->load($include)));
|
|
|
|
return $this->respondWithDocument($document);
|
|
}
|
|
}
|