mirror of
https://github.com/flarum/framework.git
synced 2025-07-13 21:05:27 +08:00
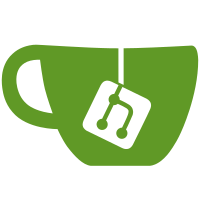
- An API action handles a Flarum\Api\Request, which is a simple object containing an array of params, the actor, and optionally an HTTP request object - Most API actions use SerializeAction as a base, which parses request input according to the JSON-API spec (creates a JsonApiRequest object), runs the child class method to get data, then serializes it and assigns it to a JsonApiResponse (standard HTTP response with a Tobscure\JsonApi\Document as content) - The JSON-API request input parsing is subject to restrictions as defined by public static properties on the action (i.e. extensible) - Also the actor is given to the serializer instance now, instead of being a static property
41 lines
979 B
PHP
41 lines
979 B
PHP
<?php namespace Flarum\Api\Actions\Discussions;
|
|
|
|
use Flarum\Core\Commands\DeleteDiscussionCommand;
|
|
use Flarum\Api\Actions\DeleteAction as BaseDeleteAction;
|
|
use Flarum\Api\Request;
|
|
use Illuminate\Http\Response;
|
|
use Illuminate\Contracts\Bus\Dispatcher;
|
|
|
|
class DeleteAction extends BaseDeleteAction
|
|
{
|
|
/**
|
|
* The command bus.
|
|
*
|
|
* @var \Illuminate\Contracts\Bus\Dispatcher
|
|
*/
|
|
protected $bus;
|
|
|
|
/**
|
|
* Initialize the action.
|
|
*
|
|
* @param \Illuminate\Contracts\Bus\Dispatcher $bus
|
|
*/
|
|
public function __construct(Dispatcher $bus)
|
|
{
|
|
$this->bus = $bus;
|
|
}
|
|
|
|
/**
|
|
* Delete a discussion according to input from the API request.
|
|
*
|
|
* @param \Flarum\Api\Request $request
|
|
* @return void
|
|
*/
|
|
protected function delete(Request $request, Response $response)
|
|
{
|
|
$this->bus->dispatch(
|
|
new DeleteDiscussionCommand($request->get('id'), $request->actor->getUser())
|
|
);
|
|
}
|
|
}
|