mirror of
https://github.com/flarum/framework.git
synced 2025-05-22 06:39:57 +08:00
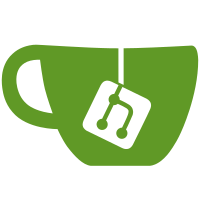
- Use contextual namespaces within Flarum\Core - Clean up and docblock everything - Refactor Activity/Notification blueprint stuff - Refactor Formatter stuff - Refactor Search stuff - Upgrade to JSON-API 1.0 - Removed “addedPosts” and “removedPosts” relationships from discussion API. This was used for adding/removing event posts after renaming a discussion etc. Instead we should make an additional request to get all new posts Todo: - Fix Extenders and extensions - Get rid of repository interfaces - Fix other bugs I’ve inevitably introduced
99 lines
2.9 KiB
JavaScript
99 lines
2.9 KiB
JavaScript
import ItemList from 'flarum/utils/item-list';
|
|
import ComposerBody from 'flarum/components/composer-body';
|
|
import Alert from 'flarum/components/alert';
|
|
import ActionButton from 'flarum/components/action-button';
|
|
import Composer from 'flarum/components/composer';
|
|
import icon from 'flarum/helpers/icon';
|
|
|
|
export default class ReplyComposer extends ComposerBody {
|
|
constructor(props) {
|
|
props.placeholder = props.placeholder || 'Write a Reply...';
|
|
props.submitLabel = props.submitLabel || 'Post Reply';
|
|
props.confirmExit = props.confirmExit || 'You have not posted your reply. Do you wish to discard it?';
|
|
|
|
super(props);
|
|
}
|
|
|
|
view() {
|
|
return super.view('reply-composer');
|
|
}
|
|
|
|
headerItems() {
|
|
var items = new ItemList();
|
|
|
|
items.add('title', m('h3', [
|
|
icon('reply'), ' ',
|
|
m('a', {href: app.route.discussion(this.props.discussion), config: m.route}, this.props.discussion.title())
|
|
]));
|
|
|
|
return items;
|
|
}
|
|
|
|
data() {
|
|
return {
|
|
content: this.content(),
|
|
relationships: {discussion: this.props.discussion}
|
|
};
|
|
}
|
|
|
|
onsubmit() {
|
|
var discussion = this.props.discussion;
|
|
|
|
this.loading(true);
|
|
m.redraw();
|
|
|
|
var data = this.data();
|
|
|
|
app.store.createRecord('posts').save(data).then((post) => {
|
|
app.composer.hide();
|
|
|
|
discussion.pushAttributes({
|
|
relationships: {
|
|
lastUser: post.user(),
|
|
lastPost: post
|
|
},
|
|
lastTime: post.time(),
|
|
lastPostNumber: post.number(),
|
|
commentsCount: discussion.commentsCount() + 1,
|
|
readTime: post.time(),
|
|
readNumber: post.number()
|
|
});
|
|
discussion.data().relationships.posts.data.push({type: 'posts', id: post.id()});
|
|
|
|
// If we're currently viewing the discussion which this reply was made
|
|
// in, then we can add the post to the end of the post stream.
|
|
if (app.current && app.current.discussion && app.current.discussion().id() === discussion.id()) {
|
|
app.current.stream.pushPost(post);
|
|
m.route(app.route('discussion.near', {
|
|
id: discussion.id(),
|
|
slug: discussion.slug(),
|
|
near: post.number()
|
|
}));
|
|
} else {
|
|
// Otherwise, we'll create an alert message to inform the user that
|
|
// their reply has been posted, containing a button which will
|
|
// transition to their new post when clicked.
|
|
var alert;
|
|
var viewButton = ActionButton.component({
|
|
label: 'View',
|
|
onclick: () => {
|
|
m.route(app.route('discussion.near', { id: discussion.id(), slug: discussion.slug(), near: post.number() }));
|
|
app.alerts.dismiss(alert);
|
|
}
|
|
});
|
|
app.alerts.show(
|
|
alert = new Alert({
|
|
type: 'success',
|
|
message: 'Your reply was posted.',
|
|
controls: [viewButton]
|
|
})
|
|
);
|
|
}
|
|
}, errors => {
|
|
this.loading(false);
|
|
m.redraw();
|
|
app.handleApiErrors(errors);
|
|
});
|
|
}
|
|
}
|