mirror of
https://github.com/flarum/framework.git
synced 2025-05-23 23:29:57 +08:00
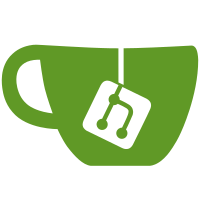
Improved consistency for existing core translation key names. See flarum/core#265 - Completely overhauled core en.yml - Replaced existing key names in all core JS files to match - Extracted a hardcoded string in IndexPage.js - Combined two app.trans calls in DiscussionControls.js - Removed hardcoded spaces from LogInModal.js and SignUpModal.js - Added two new keys from DiscussionControls.js (soft delete) - Created two new “reused keys” to YML to accommodate same
105 lines
2.2 KiB
JavaScript
105 lines
2.2 KiB
JavaScript
import Component from 'flarum/Component';
|
|
import LoadingIndicator from 'flarum/components/LoadingIndicator';
|
|
import classList from 'flarum/utils/classList';
|
|
|
|
/**
|
|
* The `UserBio` component displays a user's bio, optionally letting the user
|
|
* edit it.
|
|
*/
|
|
export default class UserBio extends Component {
|
|
constructor(...args) {
|
|
super(...args);
|
|
|
|
/**
|
|
* Whether or not the bio is currently being edited.
|
|
*
|
|
* @type {Boolean}
|
|
*/
|
|
this.editing = false;
|
|
|
|
/**
|
|
* Whether or not the bio is currently being saved.
|
|
*
|
|
* @type {Boolean}
|
|
*/
|
|
this.loading = false;
|
|
}
|
|
|
|
view() {
|
|
const user = this.props.user;
|
|
let content;
|
|
|
|
if (this.editing) {
|
|
content = <textarea className="FormControl" placeholder={app.trans('core.user_bio_placeholder')} rows="3" value={user.bio()}/>;
|
|
} else {
|
|
let subContent;
|
|
|
|
if (this.loading) {
|
|
subContent = <p className="UserBio-placeholder">{LoadingIndicator.component({size: 'tiny'})}</p>;
|
|
} else {
|
|
const bioHtml = user.bioHtml();
|
|
|
|
if (bioHtml) {
|
|
subContent = m.trust(bioHtml);
|
|
} else if (this.props.editable) {
|
|
subContent = <p className="UserBio-placeholder">{app.trans('core.user_bio_placeholder')}</p>;
|
|
}
|
|
}
|
|
|
|
content = <div className="UserBio-content">{subContent}</div>;
|
|
}
|
|
|
|
return (
|
|
<div className={'UserBio ' + classList({
|
|
editable: this.props.editable,
|
|
editing: this.editing
|
|
})}
|
|
onclick={this.edit.bind(this)}>
|
|
{content}
|
|
</div>
|
|
);
|
|
}
|
|
|
|
/**
|
|
* Edit the bio.
|
|
*/
|
|
edit() {
|
|
if (!this.props.editable) return;
|
|
|
|
this.editing = true;
|
|
m.redraw();
|
|
|
|
const bio = this;
|
|
const save = function(e) {
|
|
if (e.shiftKey) return;
|
|
e.preventDefault();
|
|
bio.save($(this).val());
|
|
};
|
|
|
|
this.$('textarea').focus()
|
|
.bind('blur', save)
|
|
.bind('keydown', 'return', save);
|
|
}
|
|
|
|
/**
|
|
* Save the bio.
|
|
*
|
|
* @param {String} value
|
|
*/
|
|
save(value) {
|
|
const user = this.props.user;
|
|
|
|
if (user.bio() !== value) {
|
|
this.loading = true;
|
|
|
|
user.save({bio: value}).then(() => {
|
|
this.loading = false;
|
|
m.redraw();
|
|
});
|
|
}
|
|
|
|
this.editing = false;
|
|
m.redraw();
|
|
}
|
|
}
|