mirror of
https://github.com/rclone/rclone.git
synced 2025-04-19 10:08:56 +08:00
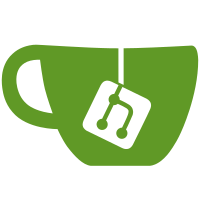
Some checks failed
build / windows (push) Has been cancelled
build / other_os (push) Has been cancelled
build / mac_amd64 (push) Has been cancelled
build / mac_arm64 (push) Has been cancelled
build / linux (push) Has been cancelled
build / go1.23 (push) Has been cancelled
build / linux_386 (push) Has been cancelled
build / lint (push) Has been cancelled
build / android-all (push) Has been cancelled
Build & Push Docker Images / Build Docker Image for linux/arm/v6 (push) Has been cancelled
Build & Push Docker Images / Build Docker Image for linux/arm/v7 (push) Has been cancelled
Build & Push Docker Images / Build Docker Image for linux/arm64 (push) Has been cancelled
Build & Push Docker Images / Build Docker Image for linux/386 (push) Has been cancelled
Build & Push Docker Images / Build Docker Image for linux/amd64 (push) Has been cancelled
Build & Push Docker Images / Merge & Push Final Docker Image (push) Has been cancelled
This change adds a new vendor called "infinitescale" to the webdav backend. It enables the ownCloud Infinite Scale https://github.com/owncloud/ocis project and implements its specific chunked uploader following the tus protocol https://tus.io Signed-off-by: Christian Richter <crichter@owncloud.com> Co-authored-by: Klaas Freitag <klaas.freitag@kiteworks.com> Co-authored-by: Christian Richter <crichter@owncloud.com> Co-authored-by: Christian Richter <1058116+dragonchaser@users.noreply.github.com> Co-authored-by: Ralf Haferkamp <r.haferkamp@opencloud.eu>
41 lines
1.5 KiB
Go
41 lines
1.5 KiB
Go
package webdav
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
)
|
|
|
|
var (
|
|
// ErrChunkSize is returned when the chunk size is zero
|
|
ErrChunkSize = errors.New("tus chunk size must be greater than zero")
|
|
// ErrNilLogger is returned when the logger is nil
|
|
ErrNilLogger = errors.New("tus logger can't be nil")
|
|
// ErrNilStore is returned when the store is nil
|
|
ErrNilStore = errors.New("tus store can't be nil if resume is enable")
|
|
// ErrNilUpload is returned when the upload is nil
|
|
ErrNilUpload = errors.New("tus upload can't be nil")
|
|
// ErrLargeUpload is returned when the upload body is to large
|
|
ErrLargeUpload = errors.New("tus upload body is to large")
|
|
// ErrVersionMismatch is returned when the tus protocol version is mismatching
|
|
ErrVersionMismatch = errors.New("tus protocol version mismatch")
|
|
// ErrOffsetMismatch is returned when the tus upload offset is mismatching
|
|
ErrOffsetMismatch = errors.New("tus upload offset mismatch")
|
|
// ErrUploadNotFound is returned when the tus upload is not found
|
|
ErrUploadNotFound = errors.New("tus upload not found")
|
|
// ErrResumeNotEnabled is returned when the tus resuming is not enabled
|
|
ErrResumeNotEnabled = errors.New("tus resuming not enabled")
|
|
// ErrFingerprintNotSet is returned when the tus fingerprint is not set
|
|
ErrFingerprintNotSet = errors.New("tus fingerprint not set")
|
|
)
|
|
|
|
// ClientError represents an error state of a client
|
|
type ClientError struct {
|
|
Code int
|
|
Body []byte
|
|
}
|
|
|
|
// Error returns an error string containing the client error code
|
|
func (c ClientError) Error() string {
|
|
return fmt.Sprintf("unexpected status code: %d", c.Code)
|
|
}
|