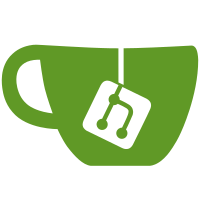
The debug assertion that asserts that services are destroyed only on the main worker would be triggered on shutdown as there is no current worker at that point in time. In addition to this, it is wrong to call service_destroy at shutdown as that will remove persisted configurations. The service_free function can be called directly as we know no other thread are running when the services are being torn down. Also added the missing check that the destroyInstance function is implemented before calling it.
65 lines
1.1 KiB
C++
65 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2016 MariaDB Corporation Ab
|
|
*
|
|
* Use of this software is governed by the Business Source License included
|
|
* in the LICENSE.TXT file and at www.mariadb.com/bsl11.
|
|
*
|
|
* Change Date: 2022-01-01
|
|
*
|
|
* On the date above, in accordance with the Business Source License, use
|
|
* of this software will be governed by version 2 or later of the General
|
|
* Public License.
|
|
*/
|
|
|
|
#include <maxscale/maxscale.h>
|
|
|
|
#include <time.h>
|
|
|
|
#include "internal/maxscale.h"
|
|
#include "internal/routingworker.hh"
|
|
#include "internal/service.hh"
|
|
|
|
static time_t started;
|
|
|
|
void maxscale_reset_starttime(void)
|
|
{
|
|
started = time(0);
|
|
}
|
|
|
|
time_t maxscale_started(void)
|
|
{
|
|
return started;
|
|
}
|
|
|
|
int maxscale_uptime()
|
|
{
|
|
return time(0) - started;
|
|
}
|
|
|
|
int maxscale_shutdown()
|
|
{
|
|
static int n_shutdowns = 0;
|
|
|
|
int n = atomic_add(&n_shutdowns, 1);
|
|
|
|
if (n == 0)
|
|
{
|
|
service_shutdown();
|
|
mxs::RoutingWorker::shutdown_all();
|
|
}
|
|
|
|
return n + 1;
|
|
}
|
|
|
|
static bool teardown_in_progress = false;
|
|
|
|
bool maxscale_teardown_in_progress()
|
|
{
|
|
return teardown_in_progress;
|
|
}
|
|
|
|
void maxscale_start_teardown()
|
|
{
|
|
teardown_in_progress = true;
|
|
}
|