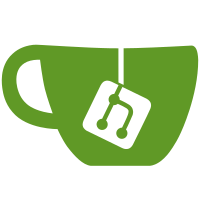
The code in avrorouter that returned the current transaction was not very useful and it can be acquired via the REST API in a more convenient format. The number of created sessions is tracked on the service level so there is no need to track it in the avrorouter. Removed declarations for functions that do not exist and moved code around to reduce the scope.
195 lines
6.0 KiB
C++
195 lines
6.0 KiB
C++
/*
|
|
* Copyright (c) 2016 MariaDB Corporation Ab
|
|
*
|
|
* Use of this software is governed by the Business Source License included
|
|
* in the LICENSE.TXT file and at www.mariadb.com/bsl11.
|
|
*
|
|
* Change Date: 2020-01-01
|
|
*
|
|
* On the date above, in accordance with the Business Source License, use
|
|
* of this software will be governed by version 2 or later of the General
|
|
* Public License.
|
|
*/
|
|
|
|
/**
|
|
* @file avro_index.c - GTID to file position index
|
|
*
|
|
* This file contains functions used to store index information
|
|
* about GTID position in an Avro file. Since all records in the Avro file
|
|
* that avrorouter uses contain the common GTID field, we can use it to create
|
|
* an index. This can then be used to speed up retrieval of Avro records by
|
|
* seeking to the offset of the file and reading the record instead of iterating
|
|
* through all the records and looking for a matching record.
|
|
*
|
|
* The index is stored as an SQLite3 database.
|
|
*/
|
|
|
|
#include "avrorouter.hh"
|
|
|
|
#include <maxscale/debug.h>
|
|
#include <glob.h>
|
|
|
|
static const char insert_template[] = "INSERT INTO gtid(domain, server_id, "
|
|
"sequence, avrofile, position) values (%lu, %lu, %lu, \"%s\", %ld);";
|
|
|
|
static void set_gtid(gtid_pos_t *gtid, json_t *row)
|
|
{
|
|
json_t *obj = json_object_get(row, avro_sequence);
|
|
ss_dassert(json_is_integer(obj));
|
|
gtid->seq = json_integer_value(obj);
|
|
|
|
obj = json_object_get(row, avro_server_id);
|
|
ss_dassert(json_is_integer(obj));
|
|
gtid->server_id = json_integer_value(obj);
|
|
|
|
obj = json_object_get(row, avro_domain);
|
|
ss_dassert(json_is_integer(obj));
|
|
gtid->domain = json_integer_value(obj);
|
|
}
|
|
|
|
int index_query_cb(void *data, int rows, char** values, char** names)
|
|
{
|
|
for (int i = 0; i < rows; i++)
|
|
{
|
|
if (values[i])
|
|
{
|
|
*((long*)data) = strtol(values[i], NULL, 10);
|
|
return 0;
|
|
}
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
void avro_index_file(Avro *router, const char* filename)
|
|
{
|
|
MAXAVRO_FILE *file = maxavro_file_open(filename);
|
|
|
|
if (file)
|
|
{
|
|
const char *name = strrchr(filename, '/');
|
|
ss_dassert(name);
|
|
|
|
if (name)
|
|
{
|
|
char sql[AVRO_SQL_BUFFER_SIZE];
|
|
char *errmsg;
|
|
long pos = -1;
|
|
name++;
|
|
|
|
snprintf(sql, sizeof(sql), "SELECT position FROM " INDEX_TABLE_NAME
|
|
" WHERE filename=\"%s\";", name);
|
|
|
|
if (sqlite3_exec(router->sqlite_handle, sql, index_query_cb, &pos, &errmsg) != SQLITE_OK)
|
|
{
|
|
MXS_ERROR("Failed to read last indexed position of file '%s': %s",
|
|
name, errmsg);
|
|
sqlite3_free(errmsg);
|
|
maxavro_file_close(file);
|
|
return;
|
|
}
|
|
|
|
/** Continue from last position */
|
|
if (pos > 0 && !maxavro_record_set_pos(file, pos))
|
|
{
|
|
maxavro_file_close(file);
|
|
return;
|
|
}
|
|
|
|
gtid_pos_t prev_gtid;
|
|
|
|
if (sqlite3_exec(router->sqlite_handle, "BEGIN", NULL, NULL, &errmsg) != SQLITE_OK)
|
|
{
|
|
MXS_ERROR("Failed to start transaction: %s", errmsg);
|
|
}
|
|
sqlite3_free(errmsg);
|
|
|
|
do
|
|
{
|
|
json_t *row = maxavro_record_read_json(file);
|
|
|
|
if (row)
|
|
{
|
|
gtid_pos_t gtid;
|
|
set_gtid(>id, row);
|
|
|
|
if (prev_gtid.domain != gtid.domain ||
|
|
prev_gtid.server_id != gtid.server_id ||
|
|
prev_gtid.seq != gtid.seq)
|
|
{
|
|
snprintf(sql, sizeof(sql), insert_template, gtid.domain,
|
|
gtid.server_id, gtid.seq, name, file->block_start_pos);
|
|
if (sqlite3_exec(router->sqlite_handle, sql, NULL, NULL,
|
|
&errmsg) != SQLITE_OK)
|
|
{
|
|
MXS_ERROR("Failed to insert GTID %lu-%lu-%lu for %s "
|
|
"into index database: %s", gtid.domain,
|
|
gtid.server_id, gtid.seq, name, errmsg);
|
|
|
|
}
|
|
sqlite3_free(errmsg);
|
|
errmsg = NULL;
|
|
prev_gtid = gtid;
|
|
}
|
|
json_decref(row);
|
|
}
|
|
else
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
while (maxavro_next_block(file));
|
|
|
|
if (sqlite3_exec(router->sqlite_handle, "COMMIT", NULL, NULL, &errmsg) != SQLITE_OK)
|
|
{
|
|
MXS_ERROR("Failed to commit transaction: %s", errmsg);
|
|
}
|
|
sqlite3_free(errmsg);
|
|
|
|
snprintf(sql, sizeof(sql), "INSERT OR REPLACE INTO " INDEX_TABLE_NAME
|
|
" values (%lu, \"%s\");", file->block_start_pos, name);
|
|
if (sqlite3_exec(router->sqlite_handle, sql, NULL, NULL,
|
|
&errmsg) != SQLITE_OK)
|
|
{
|
|
MXS_ERROR("Failed to update indexing progress: %s", errmsg);
|
|
}
|
|
sqlite3_free(errmsg);
|
|
errmsg = NULL;
|
|
}
|
|
else
|
|
{
|
|
MXS_ERROR("Malformed filename: %s", filename);
|
|
}
|
|
|
|
maxavro_file_close(file);
|
|
}
|
|
else
|
|
{
|
|
MXS_ERROR("Failed to open file '%s' when generating file index.", filename);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @brief Avro file indexing task
|
|
*
|
|
* Builds an index of filenames, GTIDs and positions in the Avro file.
|
|
* This allows all tables that contain a GTID to be fetched in an effiecent
|
|
* manner.
|
|
* @param data The router instance
|
|
*/
|
|
void avro_update_index(Avro* router)
|
|
{
|
|
char path[PATH_MAX + 1];
|
|
snprintf(path, sizeof(path), "%s/*.avro", router->avrodir.c_str());
|
|
glob_t files;
|
|
|
|
if (glob(path, 0, NULL, &files) != GLOB_NOMATCH)
|
|
{
|
|
for (size_t i = 0; i < files.gl_pathc; i++)
|
|
{
|
|
avro_index_file(router, files.gl_pathv[i]);
|
|
}
|
|
}
|
|
|
|
globfree(&files);
|
|
}
|