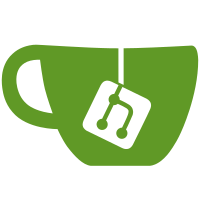
The id has now been moved from mxs::Worker to mxs::RoutingWorker and the implications are felt in many places. The primary need for the id was to be able to access worker specfic data, maintained outside of a routing worker, when given a worker (the id is used to index into an array). Slightly related to that was the need to be able to iterate over all workers. That obviously implies some kind of collection. That causes all sorts of issues if there is a need for being able to create and destroy a worker at runtime. With the id removed from mxs::Worker all those issues are gone, and its perfectly ok to create and destory mxs::Workers as needed. Further, while there is a need to broadcast a particular message to all _routing_ workers, it hardly makes sense to broadcast a particular message too _all_ workers. Consequently, only routing workers are kept in a collection and all static member functions dealing with all workers (e.g. broadcast) have now been moved to mxs::RoutingWorker. Now, instead of passing the id around we instead deal directly with the worker pointer. Later the data in all those external arrays will be moved into mxs::[Worker|RoutingWorker] so that worker related data is maintained in exactly one place.
53 lines
961 B
C++
53 lines
961 B
C++
/*
|
|
* Copyright (c) 2016 MariaDB Corporation Ab
|
|
*
|
|
* Use of this software is governed by the Business Source License included
|
|
* in the LICENSE.TXT file and at www.mariadb.com/bsl11.
|
|
*
|
|
* Change Date: 2022-01-01
|
|
*
|
|
* On the date above, in accordance with the Business Source License, use
|
|
* of this software will be governed by version 2 or later of the General
|
|
* Public License.
|
|
*/
|
|
|
|
#include <maxscale/maxscale.h>
|
|
|
|
#include <time.h>
|
|
|
|
#include "internal/maxscale.h"
|
|
#include "internal/routingworker.hh"
|
|
#include "internal/service.h"
|
|
|
|
static time_t started;
|
|
|
|
void maxscale_reset_starttime(void)
|
|
{
|
|
started = time(0);
|
|
}
|
|
|
|
time_t maxscale_started(void)
|
|
{
|
|
return started;
|
|
}
|
|
|
|
int maxscale_uptime()
|
|
{
|
|
return time(0) - started;
|
|
}
|
|
|
|
int maxscale_shutdown()
|
|
{
|
|
static int n_shutdowns = 0;
|
|
|
|
int n = atomic_add(&n_shutdowns, 1);
|
|
|
|
if (n == 0)
|
|
{
|
|
service_shutdown();
|
|
mxs::RoutingWorker::shutdown_all();
|
|
}
|
|
|
|
return n + 1;
|
|
}
|