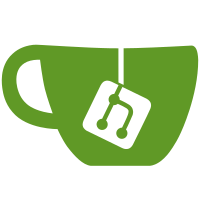
* chore: upgrade go releaser to v1.13.1 (#464) * chore: update goreleaser and actually use go 1.19 * chore: format for go 1.19 Co-authored-by: Brandon Pfeifer <bpfeifer@influxdata.com> Co-authored-by: Brandon Pfeifer <bpfeifer@influxdata.com>
2989 lines
102 KiB
Go
2989 lines
102 KiB
Go
/*
|
|
* Subset of Influx API covered by Influx CLI
|
|
*
|
|
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
|
|
*
|
|
* API version: 2.0.0
|
|
*/
|
|
|
|
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
|
|
|
|
package api
|
|
|
|
import (
|
|
_context "context"
|
|
_fmt "fmt"
|
|
_io "io"
|
|
_nethttp "net/http"
|
|
_neturl "net/url"
|
|
"strings"
|
|
"time"
|
|
)
|
|
|
|
// Linger please
|
|
var (
|
|
_ _context.Context
|
|
)
|
|
|
|
type TasksApi interface {
|
|
|
|
/*
|
|
* DeleteTasksID Delete a task
|
|
* Deletes a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) and associated records.
|
|
|
|
Use this endpoint to delete a task and all associated records (task runs, logs, and labels).
|
|
Once the task is deleted, InfluxDB cancels all scheduled runs of the task.
|
|
|
|
If you want to disable a task instead of delete it, [update the task status to `inactive`](#operation/PatchTasksID).
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to delete.
|
|
* @return ApiDeleteTasksIDRequest
|
|
*/
|
|
DeleteTasksID(ctx _context.Context, taskID string) ApiDeleteTasksIDRequest
|
|
|
|
/*
|
|
* DeleteTasksIDExecute executes the request
|
|
*/
|
|
DeleteTasksIDExecute(r ApiDeleteTasksIDRequest) error
|
|
|
|
/*
|
|
* DeleteTasksIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
*/
|
|
DeleteTasksIDExecuteWithHttpInfo(r ApiDeleteTasksIDRequest) (*_nethttp.Response, error)
|
|
|
|
/*
|
|
* DeleteTasksIDRunsID Cancel a running task
|
|
* Cancels a running [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
Use this endpoint with InfluxDB OSS to cancel a running task.
|
|
|
|
#### InfluxDB Cloud
|
|
|
|
- Doesn't support this operation.
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID The ID of the task to cancel.
|
|
* @param runID The ID of the task run to cancel.
|
|
* @return ApiDeleteTasksIDRunsIDRequest
|
|
*/
|
|
DeleteTasksIDRunsID(ctx _context.Context, taskID string, runID string) ApiDeleteTasksIDRunsIDRequest
|
|
|
|
/*
|
|
* DeleteTasksIDRunsIDExecute executes the request
|
|
*/
|
|
DeleteTasksIDRunsIDExecute(r ApiDeleteTasksIDRunsIDRequest) error
|
|
|
|
/*
|
|
* DeleteTasksIDRunsIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
*/
|
|
DeleteTasksIDRunsIDExecuteWithHttpInfo(r ApiDeleteTasksIDRunsIDRequest) (*_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetTasks List all tasks
|
|
* Retrieves a list of [tasks]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
To limit which tasks are returned, pass query parameters in your request.
|
|
If no query parameters are passed, InfluxDB returns all tasks up to the default `limit`.
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiGetTasksRequest
|
|
*/
|
|
GetTasks(ctx _context.Context) ApiGetTasksRequest
|
|
|
|
/*
|
|
* GetTasksExecute executes the request
|
|
* @return Tasks
|
|
*/
|
|
GetTasksExecute(r ApiGetTasksRequest) (Tasks, error)
|
|
|
|
/*
|
|
* GetTasksExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Tasks
|
|
*/
|
|
GetTasksExecuteWithHttpInfo(r ApiGetTasksRequest) (Tasks, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetTasksID Retrieve a task
|
|
* Retrieves a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to retrieve.
|
|
* @return ApiGetTasksIDRequest
|
|
*/
|
|
GetTasksID(ctx _context.Context, taskID string) ApiGetTasksIDRequest
|
|
|
|
/*
|
|
* GetTasksIDExecute executes the request
|
|
* @return Task
|
|
*/
|
|
GetTasksIDExecute(r ApiGetTasksIDRequest) (Task, error)
|
|
|
|
/*
|
|
* GetTasksIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Task
|
|
*/
|
|
GetTasksIDExecuteWithHttpInfo(r ApiGetTasksIDRequest) (Task, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetTasksIDLogs Retrieve all logs for a task
|
|
* Retrieves a list of all logs for a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
When an InfluxDB task runs, a “run” record is created in the task’s history.
|
|
Logs associated with each run provide relevant log messages, timestamps, and the exit status of the run attempt.
|
|
|
|
Use this endpoint to retrieve only the log events for a task,
|
|
without additional task metadata.
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID The task ID.
|
|
* @return ApiGetTasksIDLogsRequest
|
|
*/
|
|
GetTasksIDLogs(ctx _context.Context, taskID string) ApiGetTasksIDLogsRequest
|
|
|
|
/*
|
|
* GetTasksIDLogsExecute executes the request
|
|
* @return Logs
|
|
*/
|
|
GetTasksIDLogsExecute(r ApiGetTasksIDLogsRequest) (Logs, error)
|
|
|
|
/*
|
|
* GetTasksIDLogsExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Logs
|
|
*/
|
|
GetTasksIDLogsExecuteWithHttpInfo(r ApiGetTasksIDLogsRequest) (Logs, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetTasksIDRuns List runs for a task
|
|
* Retrieves a list of runs for a [task]({{% INFLUXDB_DOCS_URL %}}/process-data/).
|
|
|
|
To limit which task runs are returned, pass query parameters in your request.
|
|
If no query parameters are passed, InfluxDB returns all task runs up to the default `limit`.
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID The ID of the task to get runs for. Only returns runs for this task.
|
|
* @return ApiGetTasksIDRunsRequest
|
|
*/
|
|
GetTasksIDRuns(ctx _context.Context, taskID string) ApiGetTasksIDRunsRequest
|
|
|
|
/*
|
|
* GetTasksIDRunsExecute executes the request
|
|
* @return Runs
|
|
*/
|
|
GetTasksIDRunsExecute(r ApiGetTasksIDRunsRequest) (Runs, error)
|
|
|
|
/*
|
|
* GetTasksIDRunsExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Runs
|
|
*/
|
|
GetTasksIDRunsExecuteWithHttpInfo(r ApiGetTasksIDRunsRequest) (Runs, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetTasksIDRunsID Retrieve a run for a task.
|
|
* Retrieves a specific run for a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
Use this endpoint to retrieve detail and logs for a specific task run.
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID The ID of the task to retrieve runs for.
|
|
* @param runID The ID of the run to retrieve.
|
|
* @return ApiGetTasksIDRunsIDRequest
|
|
*/
|
|
GetTasksIDRunsID(ctx _context.Context, taskID string, runID string) ApiGetTasksIDRunsIDRequest
|
|
|
|
/*
|
|
* GetTasksIDRunsIDExecute executes the request
|
|
* @return Run
|
|
*/
|
|
GetTasksIDRunsIDExecute(r ApiGetTasksIDRunsIDRequest) (Run, error)
|
|
|
|
/*
|
|
* GetTasksIDRunsIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Run
|
|
*/
|
|
GetTasksIDRunsIDExecuteWithHttpInfo(r ApiGetTasksIDRunsIDRequest) (Run, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetTasksIDRunsIDLogs Retrieve all logs for a run
|
|
* Retrieves all logs for a task run.
|
|
A log is a list of run events with `runID`, `time`, and `message` properties.
|
|
|
|
Use this endpoint to help analyze task performance and troubleshoot failed task runs.
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID The ID of the task to get logs for.
|
|
* @param runID The ID of the run to get logs for.
|
|
* @return ApiGetTasksIDRunsIDLogsRequest
|
|
*/
|
|
GetTasksIDRunsIDLogs(ctx _context.Context, taskID string, runID string) ApiGetTasksIDRunsIDLogsRequest
|
|
|
|
/*
|
|
* GetTasksIDRunsIDLogsExecute executes the request
|
|
* @return Logs
|
|
*/
|
|
GetTasksIDRunsIDLogsExecute(r ApiGetTasksIDRunsIDLogsRequest) (Logs, error)
|
|
|
|
/*
|
|
* GetTasksIDRunsIDLogsExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Logs
|
|
*/
|
|
GetTasksIDRunsIDLogsExecuteWithHttpInfo(r ApiGetTasksIDRunsIDLogsRequest) (Logs, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PatchTasksID Update a task
|
|
* Updates a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task),
|
|
and then cancels all scheduled runs of the task.
|
|
|
|
Use this endpoint to set, modify, or clear task properties--for example: `cron`, `name`, `flux`, `status`.
|
|
Once InfluxDB applies the update, it cancels all previously scheduled runs of the task.
|
|
|
|
To update a task, pass an object that contains the updated key-value pairs.
|
|
To activate or inactivate a task, set the `status` property.
|
|
_`"status": "inactive"`_ cancels scheduled runs and prevents manual runs of the task.
|
|
|
|
#### InfluxDB Cloud
|
|
|
|
- Use either `flux` or `scriptID` to provide the task script.
|
|
|
|
- `flux`: a string of "raw" Flux that contains task options and the script--for example:
|
|
|
|
```json
|
|
{
|
|
"flux": "option task = {name: \"CPU Total 1 Hour New\", every: 1h}\
|
|
from(bucket: \"telegraf\")
|
|
|> range(start: -1h)
|
|
|> filter(fn: (r) => (r._measurement == \"cpu\"))
|
|
|> filter(fn: (r) =>\n\t\t(r._field == \"usage_system\"))
|
|
|> filter(fn: (r) => (r.cpu == \"cpu-total\"))
|
|
|> aggregateWindow(every: 1h, fn: max)
|
|
|> to(bucket: \"cpu_usage_user_total_1h\", org: \"INFLUX_ORG\")",
|
|
"status": "active",
|
|
"description": "This task downsamples CPU data every hour"
|
|
}
|
|
```
|
|
|
|
- `scriptID`: the ID of an [invokable script](#tag/Invokable-Scripts)
|
|
for the task to run.
|
|
To pass task options when using `scriptID`, pass the options as
|
|
properties in the request body--for example:
|
|
|
|
```json
|
|
{
|
|
"name": "CPU Total 1 Hour New",
|
|
"description": "This task downsamples CPU data every hour",
|
|
"every": "1h",
|
|
"scriptID": "SCRIPT_ID",
|
|
"scriptParameters":
|
|
{
|
|
"rangeStart": "-1h",
|
|
"bucket": "telegraf",
|
|
"filterField": "cpu-total"
|
|
}
|
|
}
|
|
```
|
|
|
|
#### Limitations:
|
|
|
|
- You can't use `flux` and `scriptID` for the same task.
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to update.
|
|
* @return ApiPatchTasksIDRequest
|
|
*/
|
|
PatchTasksID(ctx _context.Context, taskID string) ApiPatchTasksIDRequest
|
|
|
|
/*
|
|
* PatchTasksIDExecute executes the request
|
|
* @return Task
|
|
*/
|
|
PatchTasksIDExecute(r ApiPatchTasksIDRequest) (Task, error)
|
|
|
|
/*
|
|
* PatchTasksIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Task
|
|
*/
|
|
PatchTasksIDExecuteWithHttpInfo(r ApiPatchTasksIDRequest) (Task, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PostTasks Create a task
|
|
* Creates a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) and returns the task.
|
|
|
|
Use this endpoint to create a scheduled task that runs a Flux script.
|
|
|
|
#### InfluxDB Cloud
|
|
|
|
- You can use either `flux` or `scriptID` to provide the task script.
|
|
|
|
- `flux`: a string of "raw" Flux that contains task options and the script--for example:
|
|
|
|
```json
|
|
{
|
|
"flux": "option task = {name: \"CPU Total 1 Hour New\", every: 1h}\
|
|
from(bucket: \"telegraf\")
|
|
|> range(start: -1h)
|
|
|> filter(fn: (r) => (r._measurement == \"cpu\"))
|
|
|> filter(fn: (r) =>\n\t\t(r._field == \"usage_system\"))
|
|
|> filter(fn: (r) => (r.cpu == \"cpu-total\"))
|
|
|> aggregateWindow(every: 1h, fn: max)
|
|
|> to(bucket: \"cpu_usage_user_total_1h\", org: \"INFLUX_ORG\")",
|
|
"status": "active",
|
|
"description": "This task downsamples CPU data every hour"
|
|
}
|
|
```
|
|
|
|
- `scriptID`: the ID of an [invokable script](#tag/Invokable-Scripts)
|
|
for the task to run.
|
|
To pass task options when using `scriptID`, pass the options as
|
|
properties in the request body--for example:
|
|
|
|
```json
|
|
{
|
|
"name": "CPU Total 1 Hour New",
|
|
"description": "This task downsamples CPU data every hour",
|
|
"every": "1h",
|
|
"scriptID": "SCRIPT_ID",
|
|
"scriptParameters":
|
|
{
|
|
"rangeStart": "-1h",
|
|
"bucket": "telegraf",
|
|
"filterField": "cpu-total"
|
|
}
|
|
}
|
|
```
|
|
|
|
#### Limitations:
|
|
|
|
- You can't use `flux` and `scriptID` for the same task.
|
|
|
|
#### Related guides
|
|
|
|
- [Get started with tasks]({{% INFLUXDB_DOCS_URL %}}/process-data/get-started/)
|
|
- [Create a task]({{% INFLUXDB_DOCS_URL %}}/process-data/manage-tasks/create-task/)
|
|
- [Common tasks]({{% INFLUXDB_DOCS_URL %}}/process-data/common-tasks/)
|
|
- [Task configuration options]({{% INFLUXDB_DOCS_URL %}}/process-data/task-options/)
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiPostTasksRequest
|
|
*/
|
|
PostTasks(ctx _context.Context) ApiPostTasksRequest
|
|
|
|
/*
|
|
* PostTasksExecute executes the request
|
|
* @return Task
|
|
*/
|
|
PostTasksExecute(r ApiPostTasksRequest) (Task, error)
|
|
|
|
/*
|
|
* PostTasksExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Task
|
|
*/
|
|
PostTasksExecuteWithHttpInfo(r ApiPostTasksRequest) (Task, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PostTasksIDRuns Start a task run, overriding the schedule
|
|
* Schedules a task run to start immediately, ignoring scheduled runs.
|
|
|
|
Use this endpoint to manually start a task run.
|
|
Scheduled runs will continue to run as scheduled.
|
|
This may result in concurrently running tasks.
|
|
|
|
To _retry_ a previous run (and avoid creating a new run),
|
|
use the [`POST /api/v2/tasks/{taskID}/runs/{runID}/retry` endpoint](#operation/PostTasksIDRunsIDRetry).
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID
|
|
* @return ApiPostTasksIDRunsRequest
|
|
*/
|
|
PostTasksIDRuns(ctx _context.Context, taskID string) ApiPostTasksIDRunsRequest
|
|
|
|
/*
|
|
* PostTasksIDRunsExecute executes the request
|
|
* @return Run
|
|
*/
|
|
PostTasksIDRunsExecute(r ApiPostTasksIDRunsRequest) (Run, error)
|
|
|
|
/*
|
|
* PostTasksIDRunsExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Run
|
|
*/
|
|
PostTasksIDRunsExecuteWithHttpInfo(r ApiPostTasksIDRunsRequest) (Run, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PostTasksIDRunsIDRetry Retry a task run
|
|
* Queues a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) run to
|
|
retry and returns the scheduled run.
|
|
|
|
To manually start a _new_ task run, use the
|
|
[`POST /api/v2/tasks/{taskID}/runs` endpoint](#operation/PostTasksIDRuns).
|
|
|
|
#### Limitations
|
|
|
|
- The task must be _active_ (`status: "active"`).
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to retry.
|
|
* @param runID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) run ID. Specifies the task run to retry. To find a task run ID, use the [`GET /api/v2/tasks/{taskID}/runs` endpoint](#operation/GetTasksIDRuns) to list task runs.
|
|
* @return ApiPostTasksIDRunsIDRetryRequest
|
|
*/
|
|
PostTasksIDRunsIDRetry(ctx _context.Context, taskID string, runID string) ApiPostTasksIDRunsIDRetryRequest
|
|
|
|
/*
|
|
* PostTasksIDRunsIDRetryExecute executes the request
|
|
* @return Run
|
|
*/
|
|
PostTasksIDRunsIDRetryExecute(r ApiPostTasksIDRunsIDRetryRequest) (Run, error)
|
|
|
|
/*
|
|
* PostTasksIDRunsIDRetryExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Run
|
|
*/
|
|
PostTasksIDRunsIDRetryExecuteWithHttpInfo(r ApiPostTasksIDRunsIDRetryRequest) (Run, *_nethttp.Response, error)
|
|
}
|
|
|
|
// TasksApiService TasksApi service
|
|
type TasksApiService service
|
|
|
|
type ApiDeleteTasksIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRequest) TaskID(taskID string) ApiDeleteTasksIDRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiDeleteTasksIDRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRequest) ZapTraceSpan(zapTraceSpan string) ApiDeleteTasksIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiDeleteTasksIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRequest) Execute() error {
|
|
return r.ApiService.DeleteTasksIDExecute(r)
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRequest) ExecuteWithHttpInfo() (*_nethttp.Response, error) {
|
|
return r.ApiService.DeleteTasksIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- DeleteTasksID Delete a task
|
|
- Deletes a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) and associated records.
|
|
|
|
Use this endpoint to delete a task and all associated records (task runs, logs, and labels).
|
|
Once the task is deleted, InfluxDB cancels all scheduled runs of the task.
|
|
|
|
If you want to disable a task instead of delete it, [update the task status to `inactive`](#operation/PatchTasksID).
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to delete.
|
|
- @return ApiDeleteTasksIDRequest
|
|
*/
|
|
func (a *TasksApiService) DeleteTasksID(ctx _context.Context, taskID string) ApiDeleteTasksIDRequest {
|
|
return ApiDeleteTasksIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
*/
|
|
func (a *TasksApiService) DeleteTasksIDExecute(r ApiDeleteTasksIDRequest) error {
|
|
_, err := a.DeleteTasksIDExecuteWithHttpInfo(r)
|
|
return err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
*/
|
|
func (a *TasksApiService) DeleteTasksIDExecuteWithHttpInfo(r ApiDeleteTasksIDRequest) (*_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodDelete
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.DeleteTasksID")
|
|
if err != nil {
|
|
return nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiDeleteTasksIDRunsIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
runID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRunsIDRequest) TaskID(taskID string) ApiDeleteTasksIDRunsIDRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiDeleteTasksIDRunsIDRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRunsIDRequest) RunID(runID string) ApiDeleteTasksIDRunsIDRequest {
|
|
r.runID = runID
|
|
return r
|
|
}
|
|
func (r ApiDeleteTasksIDRunsIDRequest) GetRunID() string {
|
|
return r.runID
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRunsIDRequest) ZapTraceSpan(zapTraceSpan string) ApiDeleteTasksIDRunsIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiDeleteTasksIDRunsIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRunsIDRequest) Execute() error {
|
|
return r.ApiService.DeleteTasksIDRunsIDExecute(r)
|
|
}
|
|
|
|
func (r ApiDeleteTasksIDRunsIDRequest) ExecuteWithHttpInfo() (*_nethttp.Response, error) {
|
|
return r.ApiService.DeleteTasksIDRunsIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- DeleteTasksIDRunsID Cancel a running task
|
|
- Cancels a running [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
Use this endpoint with InfluxDB OSS to cancel a running task.
|
|
|
|
#### InfluxDB Cloud
|
|
|
|
- Doesn't support this operation.
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID The ID of the task to cancel.
|
|
- @param runID The ID of the task run to cancel.
|
|
- @return ApiDeleteTasksIDRunsIDRequest
|
|
*/
|
|
func (a *TasksApiService) DeleteTasksIDRunsID(ctx _context.Context, taskID string, runID string) ApiDeleteTasksIDRunsIDRequest {
|
|
return ApiDeleteTasksIDRunsIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
runID: runID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
*/
|
|
func (a *TasksApiService) DeleteTasksIDRunsIDExecute(r ApiDeleteTasksIDRunsIDRequest) error {
|
|
_, err := a.DeleteTasksIDRunsIDExecuteWithHttpInfo(r)
|
|
return err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
*/
|
|
func (a *TasksApiService) DeleteTasksIDRunsIDExecuteWithHttpInfo(r ApiDeleteTasksIDRunsIDRequest) (*_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodDelete
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.DeleteTasksIDRunsID")
|
|
if err != nil {
|
|
return nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}/runs/{runID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
localVarPath = strings.Replace(localVarPath, "{"+"runID"+"}", _neturl.PathEscape(parameterToString(r.runID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
if localVarHTTPResponse.StatusCode == 405 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetTasksRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
zapTraceSpan *string
|
|
name *string
|
|
after *string
|
|
user *string
|
|
org *string
|
|
orgID *string
|
|
status *string
|
|
limit *int32
|
|
offset *int32
|
|
sortBy *string
|
|
type_ *string
|
|
scriptID *string
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) ZapTraceSpan(zapTraceSpan string) ApiGetTasksRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) Name(name string) ApiGetTasksRequest {
|
|
r.name = &name
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetName() *string {
|
|
return r.name
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) After(after string) ApiGetTasksRequest {
|
|
r.after = &after
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetAfter() *string {
|
|
return r.after
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) User(user string) ApiGetTasksRequest {
|
|
r.user = &user
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetUser() *string {
|
|
return r.user
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) Org(org string) ApiGetTasksRequest {
|
|
r.org = &org
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetOrg() *string {
|
|
return r.org
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) OrgID(orgID string) ApiGetTasksRequest {
|
|
r.orgID = &orgID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetOrgID() *string {
|
|
return r.orgID
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) Status(status string) ApiGetTasksRequest {
|
|
r.status = &status
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetStatus() *string {
|
|
return r.status
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) Limit(limit int32) ApiGetTasksRequest {
|
|
r.limit = &limit
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetLimit() *int32 {
|
|
return r.limit
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) Offset(offset int32) ApiGetTasksRequest {
|
|
r.offset = &offset
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetOffset() *int32 {
|
|
return r.offset
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) SortBy(sortBy string) ApiGetTasksRequest {
|
|
r.sortBy = &sortBy
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetSortBy() *string {
|
|
return r.sortBy
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) Type_(type_ string) ApiGetTasksRequest {
|
|
r.type_ = &type_
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetType_() *string {
|
|
return r.type_
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) ScriptID(scriptID string) ApiGetTasksRequest {
|
|
r.scriptID = &scriptID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksRequest) GetScriptID() *string {
|
|
return r.scriptID
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) Execute() (Tasks, error) {
|
|
return r.ApiService.GetTasksExecute(r)
|
|
}
|
|
|
|
func (r ApiGetTasksRequest) ExecuteWithHttpInfo() (Tasks, *_nethttp.Response, error) {
|
|
return r.ApiService.GetTasksExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- GetTasks List all tasks
|
|
- Retrieves a list of [tasks]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
To limit which tasks are returned, pass query parameters in your request.
|
|
If no query parameters are passed, InfluxDB returns all tasks up to the default `limit`.
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @return ApiGetTasksRequest
|
|
*/
|
|
func (a *TasksApiService) GetTasks(ctx _context.Context) ApiGetTasksRequest {
|
|
return ApiGetTasksRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Tasks
|
|
*/
|
|
func (a *TasksApiService) GetTasksExecute(r ApiGetTasksRequest) (Tasks, error) {
|
|
returnVal, _, err := a.GetTasksExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Tasks
|
|
*/
|
|
func (a *TasksApiService) GetTasksExecuteWithHttpInfo(r ApiGetTasksRequest) (Tasks, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Tasks
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.GetTasks")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks"
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
if r.name != nil {
|
|
localVarQueryParams.Add("name", parameterToString(*r.name, ""))
|
|
}
|
|
if r.after != nil {
|
|
localVarQueryParams.Add("after", parameterToString(*r.after, ""))
|
|
}
|
|
if r.user != nil {
|
|
localVarQueryParams.Add("user", parameterToString(*r.user, ""))
|
|
}
|
|
if r.org != nil {
|
|
localVarQueryParams.Add("org", parameterToString(*r.org, ""))
|
|
}
|
|
if r.orgID != nil {
|
|
localVarQueryParams.Add("orgID", parameterToString(*r.orgID, ""))
|
|
}
|
|
if r.status != nil {
|
|
localVarQueryParams.Add("status", parameterToString(*r.status, ""))
|
|
}
|
|
if r.limit != nil {
|
|
localVarQueryParams.Add("limit", parameterToString(*r.limit, ""))
|
|
}
|
|
if r.offset != nil {
|
|
localVarQueryParams.Add("offset", parameterToString(*r.offset, ""))
|
|
}
|
|
if r.sortBy != nil {
|
|
localVarQueryParams.Add("sortBy", parameterToString(*r.sortBy, ""))
|
|
}
|
|
if r.type_ != nil {
|
|
localVarQueryParams.Add("type", parameterToString(*r.type_, ""))
|
|
}
|
|
if r.scriptID != nil {
|
|
localVarQueryParams.Add("scriptID", parameterToString(*r.scriptID, ""))
|
|
}
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetTasksIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiGetTasksIDRequest) TaskID(taskID string) ApiGetTasksIDRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiGetTasksIDRequest) ZapTraceSpan(zapTraceSpan string) ApiGetTasksIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetTasksIDRequest) Execute() (Task, error) {
|
|
return r.ApiService.GetTasksIDExecute(r)
|
|
}
|
|
|
|
func (r ApiGetTasksIDRequest) ExecuteWithHttpInfo() (Task, *_nethttp.Response, error) {
|
|
return r.ApiService.GetTasksIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* GetTasksID Retrieve a task
|
|
* Retrieves a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to retrieve.
|
|
* @return ApiGetTasksIDRequest
|
|
*/
|
|
func (a *TasksApiService) GetTasksID(ctx _context.Context, taskID string) ApiGetTasksIDRequest {
|
|
return ApiGetTasksIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Task
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDExecute(r ApiGetTasksIDRequest) (Task, error) {
|
|
returnVal, _, err := a.GetTasksIDExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Task
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDExecuteWithHttpInfo(r ApiGetTasksIDRequest) (Task, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Task
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.GetTasksID")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetTasksIDLogsRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiGetTasksIDLogsRequest) TaskID(taskID string) ApiGetTasksIDLogsRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDLogsRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiGetTasksIDLogsRequest) ZapTraceSpan(zapTraceSpan string) ApiGetTasksIDLogsRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDLogsRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetTasksIDLogsRequest) Execute() (Logs, error) {
|
|
return r.ApiService.GetTasksIDLogsExecute(r)
|
|
}
|
|
|
|
func (r ApiGetTasksIDLogsRequest) ExecuteWithHttpInfo() (Logs, *_nethttp.Response, error) {
|
|
return r.ApiService.GetTasksIDLogsExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- GetTasksIDLogs Retrieve all logs for a task
|
|
- Retrieves a list of all logs for a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
When an InfluxDB task runs, a “run” record is created in the task’s history.
|
|
Logs associated with each run provide relevant log messages, timestamps, and the exit status of the run attempt.
|
|
|
|
Use this endpoint to retrieve only the log events for a task,
|
|
without additional task metadata.
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID The task ID.
|
|
- @return ApiGetTasksIDLogsRequest
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDLogs(ctx _context.Context, taskID string) ApiGetTasksIDLogsRequest {
|
|
return ApiGetTasksIDLogsRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Logs
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDLogsExecute(r ApiGetTasksIDLogsRequest) (Logs, error) {
|
|
returnVal, _, err := a.GetTasksIDLogsExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Logs
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDLogsExecuteWithHttpInfo(r ApiGetTasksIDLogsRequest) (Logs, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Logs
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.GetTasksIDLogs")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}/logs"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetTasksIDRunsRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
zapTraceSpan *string
|
|
after *string
|
|
limit *int32
|
|
afterTime *time.Time
|
|
beforeTime *time.Time
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) TaskID(taskID string) ApiGetTasksIDRunsRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) ZapTraceSpan(zapTraceSpan string) ApiGetTasksIDRunsRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) After(after string) ApiGetTasksIDRunsRequest {
|
|
r.after = &after
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsRequest) GetAfter() *string {
|
|
return r.after
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) Limit(limit int32) ApiGetTasksIDRunsRequest {
|
|
r.limit = &limit
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsRequest) GetLimit() *int32 {
|
|
return r.limit
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) AfterTime(afterTime time.Time) ApiGetTasksIDRunsRequest {
|
|
r.afterTime = &afterTime
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsRequest) GetAfterTime() *time.Time {
|
|
return r.afterTime
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) BeforeTime(beforeTime time.Time) ApiGetTasksIDRunsRequest {
|
|
r.beforeTime = &beforeTime
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsRequest) GetBeforeTime() *time.Time {
|
|
return r.beforeTime
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) Execute() (Runs, error) {
|
|
return r.ApiService.GetTasksIDRunsExecute(r)
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsRequest) ExecuteWithHttpInfo() (Runs, *_nethttp.Response, error) {
|
|
return r.ApiService.GetTasksIDRunsExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- GetTasksIDRuns List runs for a task
|
|
- Retrieves a list of runs for a [task]({{% INFLUXDB_DOCS_URL %}}/process-data/).
|
|
|
|
To limit which task runs are returned, pass query parameters in your request.
|
|
If no query parameters are passed, InfluxDB returns all task runs up to the default `limit`.
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID The ID of the task to get runs for. Only returns runs for this task.
|
|
- @return ApiGetTasksIDRunsRequest
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRuns(ctx _context.Context, taskID string) ApiGetTasksIDRunsRequest {
|
|
return ApiGetTasksIDRunsRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Runs
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsExecute(r ApiGetTasksIDRunsRequest) (Runs, error) {
|
|
returnVal, _, err := a.GetTasksIDRunsExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Runs
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsExecuteWithHttpInfo(r ApiGetTasksIDRunsRequest) (Runs, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Runs
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.GetTasksIDRuns")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}/runs"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
if r.after != nil {
|
|
localVarQueryParams.Add("after", parameterToString(*r.after, ""))
|
|
}
|
|
if r.limit != nil {
|
|
localVarQueryParams.Add("limit", parameterToString(*r.limit, ""))
|
|
}
|
|
if r.afterTime != nil {
|
|
localVarQueryParams.Add("afterTime", parameterToString(*r.afterTime, ""))
|
|
}
|
|
if r.beforeTime != nil {
|
|
localVarQueryParams.Add("beforeTime", parameterToString(*r.beforeTime, ""))
|
|
}
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetTasksIDRunsIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
runID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDRequest) TaskID(taskID string) ApiGetTasksIDRunsIDRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsIDRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDRequest) RunID(runID string) ApiGetTasksIDRunsIDRequest {
|
|
r.runID = runID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsIDRequest) GetRunID() string {
|
|
return r.runID
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDRequest) ZapTraceSpan(zapTraceSpan string) ApiGetTasksIDRunsIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDRequest) Execute() (Run, error) {
|
|
return r.ApiService.GetTasksIDRunsIDExecute(r)
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDRequest) ExecuteWithHttpInfo() (Run, *_nethttp.Response, error) {
|
|
return r.ApiService.GetTasksIDRunsIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- GetTasksIDRunsID Retrieve a run for a task.
|
|
- Retrieves a specific run for a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task).
|
|
|
|
Use this endpoint to retrieve detail and logs for a specific task run.
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID The ID of the task to retrieve runs for.
|
|
- @param runID The ID of the run to retrieve.
|
|
- @return ApiGetTasksIDRunsIDRequest
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsID(ctx _context.Context, taskID string, runID string) ApiGetTasksIDRunsIDRequest {
|
|
return ApiGetTasksIDRunsIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
runID: runID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Run
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsIDExecute(r ApiGetTasksIDRunsIDRequest) (Run, error) {
|
|
returnVal, _, err := a.GetTasksIDRunsIDExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Run
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsIDExecuteWithHttpInfo(r ApiGetTasksIDRunsIDRequest) (Run, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Run
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.GetTasksIDRunsID")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}/runs/{runID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
localVarPath = strings.Replace(localVarPath, "{"+"runID"+"}", _neturl.PathEscape(parameterToString(r.runID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetTasksIDRunsIDLogsRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
runID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) TaskID(taskID string) ApiGetTasksIDRunsIDLogsRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) RunID(runID string) ApiGetTasksIDRunsIDLogsRequest {
|
|
r.runID = runID
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) GetRunID() string {
|
|
return r.runID
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) ZapTraceSpan(zapTraceSpan string) ApiGetTasksIDRunsIDLogsRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) Execute() (Logs, error) {
|
|
return r.ApiService.GetTasksIDRunsIDLogsExecute(r)
|
|
}
|
|
|
|
func (r ApiGetTasksIDRunsIDLogsRequest) ExecuteWithHttpInfo() (Logs, *_nethttp.Response, error) {
|
|
return r.ApiService.GetTasksIDRunsIDLogsExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- GetTasksIDRunsIDLogs Retrieve all logs for a run
|
|
- Retrieves all logs for a task run.
|
|
|
|
A log is a list of run events with `runID`, `time`, and `message` properties.
|
|
|
|
Use this endpoint to help analyze task performance and troubleshoot failed task runs.
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID The ID of the task to get logs for.
|
|
- @param runID The ID of the run to get logs for.
|
|
- @return ApiGetTasksIDRunsIDLogsRequest
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsIDLogs(ctx _context.Context, taskID string, runID string) ApiGetTasksIDRunsIDLogsRequest {
|
|
return ApiGetTasksIDRunsIDLogsRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
runID: runID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Logs
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsIDLogsExecute(r ApiGetTasksIDRunsIDLogsRequest) (Logs, error) {
|
|
returnVal, _, err := a.GetTasksIDRunsIDLogsExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Logs
|
|
*/
|
|
func (a *TasksApiService) GetTasksIDRunsIDLogsExecuteWithHttpInfo(r ApiGetTasksIDRunsIDLogsRequest) (Logs, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Logs
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.GetTasksIDRunsIDLogs")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}/runs/{runID}/logs"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
localVarPath = strings.Replace(localVarPath, "{"+"runID"+"}", _neturl.PathEscape(parameterToString(r.runID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPatchTasksIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
taskUpdateRequest *TaskUpdateRequest
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiPatchTasksIDRequest) TaskID(taskID string) ApiPatchTasksIDRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiPatchTasksIDRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiPatchTasksIDRequest) TaskUpdateRequest(taskUpdateRequest TaskUpdateRequest) ApiPatchTasksIDRequest {
|
|
r.taskUpdateRequest = &taskUpdateRequest
|
|
return r
|
|
}
|
|
func (r ApiPatchTasksIDRequest) GetTaskUpdateRequest() *TaskUpdateRequest {
|
|
return r.taskUpdateRequest
|
|
}
|
|
|
|
func (r ApiPatchTasksIDRequest) ZapTraceSpan(zapTraceSpan string) ApiPatchTasksIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPatchTasksIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPatchTasksIDRequest) Execute() (Task, error) {
|
|
return r.ApiService.PatchTasksIDExecute(r)
|
|
}
|
|
|
|
func (r ApiPatchTasksIDRequest) ExecuteWithHttpInfo() (Task, *_nethttp.Response, error) {
|
|
return r.ApiService.PatchTasksIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- PatchTasksID Update a task
|
|
- Updates a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task),
|
|
|
|
and then cancels all scheduled runs of the task.
|
|
|
|
Use this endpoint to set, modify, or clear task properties--for example: `cron`, `name`, `flux`, `status`.
|
|
Once InfluxDB applies the update, it cancels all previously scheduled runs of the task.
|
|
|
|
To update a task, pass an object that contains the updated key-value pairs.
|
|
To activate or inactivate a task, set the `status` property.
|
|
_`"status": "inactive"`_ cancels scheduled runs and prevents manual runs of the task.
|
|
|
|
#### InfluxDB Cloud
|
|
|
|
- Use either `flux` or `scriptID` to provide the task script.
|
|
|
|
- `flux`: a string of "raw" Flux that contains task options and the script--for example:
|
|
|
|
```json
|
|
{
|
|
"flux": "option task = {name: \"CPU Total 1 Hour New\", every: 1h}\
|
|
from(bucket: \"telegraf\")
|
|
|> range(start: -1h)
|
|
|> filter(fn: (r) => (r._measurement == \"cpu\"))
|
|
|> filter(fn: (r) =>\n\t\t(r._field == \"usage_system\"))
|
|
|> filter(fn: (r) => (r.cpu == \"cpu-total\"))
|
|
|> aggregateWindow(every: 1h, fn: max)
|
|
|> to(bucket: \"cpu_usage_user_total_1h\", org: \"INFLUX_ORG\")",
|
|
"status": "active",
|
|
"description": "This task downsamples CPU data every hour"
|
|
}
|
|
```
|
|
|
|
- `scriptID`: the ID of an [invokable script](#tag/Invokable-Scripts)
|
|
for the task to run.
|
|
To pass task options when using `scriptID`, pass the options as
|
|
properties in the request body--for example:
|
|
|
|
```json
|
|
{
|
|
"name": "CPU Total 1 Hour New",
|
|
"description": "This task downsamples CPU data every hour",
|
|
"every": "1h",
|
|
"scriptID": "SCRIPT_ID",
|
|
"scriptParameters":
|
|
{
|
|
"rangeStart": "-1h",
|
|
"bucket": "telegraf",
|
|
"filterField": "cpu-total"
|
|
}
|
|
}
|
|
```
|
|
|
|
#### Limitations:
|
|
|
|
- You can't use `flux` and `scriptID` for the same task.
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to update.
|
|
- @return ApiPatchTasksIDRequest
|
|
*/
|
|
func (a *TasksApiService) PatchTasksID(ctx _context.Context, taskID string) ApiPatchTasksIDRequest {
|
|
return ApiPatchTasksIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Task
|
|
*/
|
|
func (a *TasksApiService) PatchTasksIDExecute(r ApiPatchTasksIDRequest) (Task, error) {
|
|
returnVal, _, err := a.PatchTasksIDExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Task
|
|
*/
|
|
func (a *TasksApiService) PatchTasksIDExecuteWithHttpInfo(r ApiPatchTasksIDRequest) (Task, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPatch
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Task
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.PatchTasksID")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
if r.taskUpdateRequest == nil {
|
|
return localVarReturnValue, nil, reportError("taskUpdateRequest is required and must be specified")
|
|
}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.taskUpdateRequest
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPostTasksRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskCreateRequest *TaskCreateRequest
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiPostTasksRequest) TaskCreateRequest(taskCreateRequest TaskCreateRequest) ApiPostTasksRequest {
|
|
r.taskCreateRequest = &taskCreateRequest
|
|
return r
|
|
}
|
|
func (r ApiPostTasksRequest) GetTaskCreateRequest() *TaskCreateRequest {
|
|
return r.taskCreateRequest
|
|
}
|
|
|
|
func (r ApiPostTasksRequest) ZapTraceSpan(zapTraceSpan string) ApiPostTasksRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPostTasksRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPostTasksRequest) Execute() (Task, error) {
|
|
return r.ApiService.PostTasksExecute(r)
|
|
}
|
|
|
|
func (r ApiPostTasksRequest) ExecuteWithHttpInfo() (Task, *_nethttp.Response, error) {
|
|
return r.ApiService.PostTasksExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- PostTasks Create a task
|
|
- Creates a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) and returns the task.
|
|
|
|
Use this endpoint to create a scheduled task that runs a Flux script.
|
|
|
|
#### InfluxDB Cloud
|
|
|
|
- You can use either `flux` or `scriptID` to provide the task script.
|
|
|
|
- `flux`: a string of "raw" Flux that contains task options and the script--for example:
|
|
|
|
```json
|
|
{
|
|
"flux": "option task = {name: \"CPU Total 1 Hour New\", every: 1h}\
|
|
from(bucket: \"telegraf\")
|
|
|> range(start: -1h)
|
|
|> filter(fn: (r) => (r._measurement == \"cpu\"))
|
|
|> filter(fn: (r) =>\n\t\t(r._field == \"usage_system\"))
|
|
|> filter(fn: (r) => (r.cpu == \"cpu-total\"))
|
|
|> aggregateWindow(every: 1h, fn: max)
|
|
|> to(bucket: \"cpu_usage_user_total_1h\", org: \"INFLUX_ORG\")",
|
|
"status": "active",
|
|
"description": "This task downsamples CPU data every hour"
|
|
}
|
|
```
|
|
|
|
- `scriptID`: the ID of an [invokable script](#tag/Invokable-Scripts)
|
|
for the task to run.
|
|
To pass task options when using `scriptID`, pass the options as
|
|
properties in the request body--for example:
|
|
|
|
```json
|
|
{
|
|
"name": "CPU Total 1 Hour New",
|
|
"description": "This task downsamples CPU data every hour",
|
|
"every": "1h",
|
|
"scriptID": "SCRIPT_ID",
|
|
"scriptParameters":
|
|
{
|
|
"rangeStart": "-1h",
|
|
"bucket": "telegraf",
|
|
"filterField": "cpu-total"
|
|
}
|
|
}
|
|
```
|
|
|
|
#### Limitations:
|
|
|
|
- You can't use `flux` and `scriptID` for the same task.
|
|
|
|
#### Related guides
|
|
|
|
- [Get started with tasks]({{% INFLUXDB_DOCS_URL %}}/process-data/get-started/)
|
|
- [Create a task]({{% INFLUXDB_DOCS_URL %}}/process-data/manage-tasks/create-task/)
|
|
- [Common tasks]({{% INFLUXDB_DOCS_URL %}}/process-data/common-tasks/)
|
|
- [Task configuration options]({{% INFLUXDB_DOCS_URL %}}/process-data/task-options/)
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @return ApiPostTasksRequest
|
|
*/
|
|
func (a *TasksApiService) PostTasks(ctx _context.Context) ApiPostTasksRequest {
|
|
return ApiPostTasksRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Task
|
|
*/
|
|
func (a *TasksApiService) PostTasksExecute(r ApiPostTasksRequest) (Task, error) {
|
|
returnVal, _, err := a.PostTasksExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Task
|
|
*/
|
|
func (a *TasksApiService) PostTasksExecuteWithHttpInfo(r ApiPostTasksRequest) (Task, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPost
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Task
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.PostTasks")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks"
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
if r.taskCreateRequest == nil {
|
|
return localVarReturnValue, nil, reportError("taskCreateRequest is required and must be specified")
|
|
}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.taskCreateRequest
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPostTasksIDRunsRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
zapTraceSpan *string
|
|
runManually *RunManually
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsRequest) TaskID(taskID string) ApiPostTasksIDRunsRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiPostTasksIDRunsRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsRequest) ZapTraceSpan(zapTraceSpan string) ApiPostTasksIDRunsRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPostTasksIDRunsRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsRequest) RunManually(runManually RunManually) ApiPostTasksIDRunsRequest {
|
|
r.runManually = &runManually
|
|
return r
|
|
}
|
|
func (r ApiPostTasksIDRunsRequest) GetRunManually() *RunManually {
|
|
return r.runManually
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsRequest) Execute() (Run, error) {
|
|
return r.ApiService.PostTasksIDRunsExecute(r)
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsRequest) ExecuteWithHttpInfo() (Run, *_nethttp.Response, error) {
|
|
return r.ApiService.PostTasksIDRunsExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- PostTasksIDRuns Start a task run, overriding the schedule
|
|
- Schedules a task run to start immediately, ignoring scheduled runs.
|
|
|
|
Use this endpoint to manually start a task run.
|
|
Scheduled runs will continue to run as scheduled.
|
|
This may result in concurrently running tasks.
|
|
|
|
To _retry_ a previous run (and avoid creating a new run),
|
|
use the [`POST /api/v2/tasks/{taskID}/runs/{runID}/retry` endpoint](#operation/PostTasksIDRunsIDRetry).
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID
|
|
- @return ApiPostTasksIDRunsRequest
|
|
*/
|
|
func (a *TasksApiService) PostTasksIDRuns(ctx _context.Context, taskID string) ApiPostTasksIDRunsRequest {
|
|
return ApiPostTasksIDRunsRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Run
|
|
*/
|
|
func (a *TasksApiService) PostTasksIDRunsExecute(r ApiPostTasksIDRunsRequest) (Run, error) {
|
|
returnVal, _, err := a.PostTasksIDRunsExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Run
|
|
*/
|
|
func (a *TasksApiService) PostTasksIDRunsExecuteWithHttpInfo(r ApiPostTasksIDRunsRequest) (Run, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPost
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Run
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.PostTasksIDRuns")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}/runs"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.runManually
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPostTasksIDRunsIDRetryRequest struct {
|
|
ctx _context.Context
|
|
ApiService TasksApi
|
|
taskID string
|
|
runID string
|
|
zapTraceSpan *string
|
|
body *map[string]interface{}
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) TaskID(taskID string) ApiPostTasksIDRunsIDRetryRequest {
|
|
r.taskID = taskID
|
|
return r
|
|
}
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) GetTaskID() string {
|
|
return r.taskID
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) RunID(runID string) ApiPostTasksIDRunsIDRetryRequest {
|
|
r.runID = runID
|
|
return r
|
|
}
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) GetRunID() string {
|
|
return r.runID
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) ZapTraceSpan(zapTraceSpan string) ApiPostTasksIDRunsIDRetryRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) Body(body map[string]interface{}) ApiPostTasksIDRunsIDRetryRequest {
|
|
r.body = &body
|
|
return r
|
|
}
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) GetBody() *map[string]interface{} {
|
|
return r.body
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) Execute() (Run, error) {
|
|
return r.ApiService.PostTasksIDRunsIDRetryExecute(r)
|
|
}
|
|
|
|
func (r ApiPostTasksIDRunsIDRetryRequest) ExecuteWithHttpInfo() (Run, *_nethttp.Response, error) {
|
|
return r.ApiService.PostTasksIDRunsIDRetryExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
- PostTasksIDRunsIDRetry Retry a task run
|
|
- Queues a [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) run to
|
|
|
|
retry and returns the scheduled run.
|
|
|
|
To manually start a _new_ task run, use the
|
|
[`POST /api/v2/tasks/{taskID}/runs` endpoint](#operation/PostTasksIDRuns).
|
|
|
|
#### Limitations
|
|
|
|
- The task must be _active_ (`status: "active"`).
|
|
|
|
- @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
- @param taskID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) ID. Specifies the task to retry.
|
|
- @param runID A [task]({{% INFLUXDB_DOCS_URL %}}/reference/glossary/#task) run ID. Specifies the task run to retry. To find a task run ID, use the [`GET /api/v2/tasks/{taskID}/runs` endpoint](#operation/GetTasksIDRuns) to list task runs.
|
|
- @return ApiPostTasksIDRunsIDRetryRequest
|
|
*/
|
|
func (a *TasksApiService) PostTasksIDRunsIDRetry(ctx _context.Context, taskID string, runID string) ApiPostTasksIDRunsIDRetryRequest {
|
|
return ApiPostTasksIDRunsIDRetryRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
taskID: taskID,
|
|
runID: runID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Run
|
|
*/
|
|
func (a *TasksApiService) PostTasksIDRunsIDRetryExecute(r ApiPostTasksIDRunsIDRetryRequest) (Run, error) {
|
|
returnVal, _, err := a.PostTasksIDRunsIDRetryExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Run
|
|
*/
|
|
func (a *TasksApiService) PostTasksIDRunsIDRetryExecuteWithHttpInfo(r ApiPostTasksIDRunsIDRetryRequest) (Run, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPost
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Run
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "TasksApiService.PostTasksIDRunsIDRetry")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/tasks/{taskID}/runs/{runID}/retry"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"taskID"+"}", _neturl.PathEscape(parameterToString(r.taskID, "")), -1)
|
|
localVarPath = strings.Replace(localVarPath, "{"+"runID"+"}", _neturl.PathEscape(parameterToString(r.runID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json; charset=utf-8"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.body
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
if localVarHTTPResponse.StatusCode == 401 {
|
|
var v UnauthorizedRequestError
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|