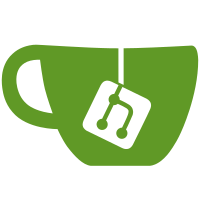
* chore: gofmt * chore: update openapi gen files * feat: add replication-bucket-name flag * fix: fix tests * chore: rename to replication-bucket * feat: show remote bucket name or id * chore: fmt fixup * chore: update openapi to master * chore: fix openapi generation
259 lines
7.6 KiB
Go
259 lines
7.6 KiB
Go
package main
|
|
|
|
import (
|
|
"github.com/influxdata/influx-cli/v2/clients/replication"
|
|
"github.com/influxdata/influx-cli/v2/pkg/cli/middleware"
|
|
"github.com/urfave/cli"
|
|
)
|
|
|
|
func newReplicationCmd() cli.Command {
|
|
return cli.Command{
|
|
Name: "replication",
|
|
Usage: "Replication stream management commands",
|
|
Before: middleware.NoArgs,
|
|
Subcommands: []cli.Command{
|
|
newReplicationCreateCmd(),
|
|
newReplicationDeleteCmd(),
|
|
newReplicationListCmd(),
|
|
newReplicationUpdateCmd(),
|
|
},
|
|
}
|
|
}
|
|
|
|
func newReplicationCreateCmd() cli.Command {
|
|
var params replication.CreateParams
|
|
return cli.Command{
|
|
Name: "create",
|
|
Usage: "Create a new replication stream",
|
|
Before: middleware.WithBeforeFns(withCli(), withApi(true), middleware.NoArgs),
|
|
Flags: append(
|
|
commonFlags(),
|
|
&cli.StringFlag{
|
|
Name: "name, n",
|
|
Usage: "Name for new replication stream",
|
|
Required: true,
|
|
Destination: ¶ms.Name,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "description, d",
|
|
Usage: "Description for new replication stream",
|
|
Destination: ¶ms.Description,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "org-id",
|
|
Usage: "The ID of the local organization",
|
|
EnvVar: "INFLUX_ORG_ID",
|
|
Destination: ¶ms.OrgID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "org, o",
|
|
Usage: "The name of the local organization",
|
|
EnvVar: "INFLUX_ORG",
|
|
Destination: ¶ms.OrgName,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "remote-id",
|
|
Usage: "Remote connection the new replication stream should send data to",
|
|
Required: true,
|
|
Destination: ¶ms.RemoteID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "local-bucket-id",
|
|
Usage: "ID of local bucket data should be replicated from",
|
|
Required: true,
|
|
Destination: ¶ms.LocalBucketID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "remote-bucket-id",
|
|
Usage: "ID of remote bucket data should be replicated to",
|
|
Destination: ¶ms.RemoteBucketID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "remote-bucket",
|
|
Usage: "Name of remote bucket data should be replicated to",
|
|
Destination: ¶ms.RemoteBucketName,
|
|
},
|
|
&cli.Int64Flag{
|
|
Name: "max-queue-bytes",
|
|
Usage: "Max queue size in bytes",
|
|
Value: 67108860, // source: https://github.com/influxdata/openapi/blob/master/src/oss/schemas/ReplicationCreationRequest.yml
|
|
Destination: ¶ms.MaxQueueSize,
|
|
},
|
|
&cli.BoolFlag{
|
|
Name: "drop-non-retryable-data",
|
|
Usage: "Drop data when a non-retryable error is encountered instead of retrying",
|
|
Destination: ¶ms.DropNonRetryableData,
|
|
},
|
|
&cli.BoolFlag{
|
|
Name: "no-drop-non-retryable-data",
|
|
Usage: "Do not drop data when a non-retryable error is encountered",
|
|
Destination: ¶ms.NoDropNonRetryableData,
|
|
},
|
|
&cli.Int64Flag{
|
|
Name: "max-age",
|
|
Usage: "Specify a maximum age (in seconds) for replications data before it is dropped, or 0 for infinite",
|
|
Value: 604800, // source: https://github.com/influxdata/openapi/blob/master/src/oss/schemas/ReplicationCreationRequest.yml
|
|
Destination: ¶ms.MaxAge,
|
|
},
|
|
),
|
|
Action: func(ctx *cli.Context) error {
|
|
api := getAPI(ctx)
|
|
|
|
client := replication.Client{
|
|
CLI: getCLI(ctx),
|
|
ReplicationsApi: api.ReplicationsApi,
|
|
OrganizationsApi: api.OrganizationsApi,
|
|
}
|
|
|
|
return client.Create(getContext(ctx), ¶ms)
|
|
},
|
|
}
|
|
}
|
|
|
|
func newReplicationDeleteCmd() cli.Command {
|
|
var replicationID string
|
|
return cli.Command{
|
|
Name: "delete",
|
|
Usage: "Delete an existing replication stream",
|
|
Before: middleware.WithBeforeFns(withCli(), withApi(true), middleware.NoArgs),
|
|
Flags: append(
|
|
commonFlags(),
|
|
&cli.StringFlag{
|
|
Name: "id, i",
|
|
Usage: "ID of the replication stream to be deleted",
|
|
Required: true,
|
|
Destination: &replicationID,
|
|
},
|
|
),
|
|
Action: func(ctx *cli.Context) error {
|
|
api := getAPI(ctx)
|
|
|
|
client := replication.Client{
|
|
CLI: getCLI(ctx),
|
|
ReplicationsApi: api.ReplicationsApi,
|
|
}
|
|
|
|
return client.Delete(getContext(ctx), replicationID)
|
|
},
|
|
}
|
|
}
|
|
|
|
func newReplicationListCmd() cli.Command {
|
|
var params replication.ListParams
|
|
return cli.Command{
|
|
Name: "list",
|
|
Usage: "List all replication streams and corresponding metrics",
|
|
Aliases: []string{"find", "ls"},
|
|
Before: middleware.WithBeforeFns(withCli(), withApi(true), middleware.NoArgs),
|
|
Flags: append(
|
|
commonFlags(),
|
|
&cli.StringFlag{
|
|
Name: "name, n",
|
|
Usage: "Filter results to only replication streams with a specific name",
|
|
Destination: ¶ms.Name,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "org-id",
|
|
Usage: "Local org ID",
|
|
EnvVar: "INFLUX_ORG_ID",
|
|
Destination: ¶ms.OrgID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "org, o",
|
|
Usage: "Local org name",
|
|
EnvVar: "INFLUX_ORG",
|
|
Destination: ¶ms.OrgName,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "remote-id",
|
|
Usage: "Filter results to only replication streams for a specific remote connection",
|
|
Destination: ¶ms.RemoteID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "local-bucket-id",
|
|
Usage: "Filter results to only replication streams for a specific local bucket",
|
|
Destination: ¶ms.LocalBucketID,
|
|
},
|
|
),
|
|
Action: func(ctx *cli.Context) error {
|
|
api := getAPI(ctx)
|
|
|
|
client := replication.Client{
|
|
CLI: getCLI(ctx),
|
|
ReplicationsApi: api.ReplicationsApi,
|
|
OrganizationsApi: api.OrganizationsApi,
|
|
}
|
|
|
|
return client.List(getContext(ctx), ¶ms)
|
|
},
|
|
}
|
|
}
|
|
|
|
func newReplicationUpdateCmd() cli.Command {
|
|
var params replication.UpdateParams
|
|
return cli.Command{
|
|
Name: "update",
|
|
Usage: "Update an existing replication stream",
|
|
Before: middleware.WithBeforeFns(withCli(), withApi(true), middleware.NoArgs),
|
|
Flags: append(
|
|
commonFlags(),
|
|
&cli.StringFlag{
|
|
Name: "id, i",
|
|
Usage: "ID of the replication stream to be updated",
|
|
Required: true,
|
|
Destination: ¶ms.ReplicationID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "name, n",
|
|
Usage: "New name for the replication stream",
|
|
Destination: ¶ms.Name,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "description, d",
|
|
Usage: "New description for the replication stream",
|
|
Destination: ¶ms.Description,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "remote-id",
|
|
Usage: "New ID of remote connection the replication stream should send data to",
|
|
Destination: ¶ms.RemoteID,
|
|
},
|
|
&cli.StringFlag{
|
|
Name: "remote-bucket-id",
|
|
Usage: "New ID of remote bucket that data should be replicated to",
|
|
Destination: ¶ms.RemoteBucketID,
|
|
},
|
|
&cli.Int64Flag{
|
|
Name: "max-queue-bytes",
|
|
Usage: "New max queue size in bytes",
|
|
Destination: ¶ms.MaxQueueSize,
|
|
},
|
|
&cli.BoolFlag{
|
|
Name: "drop-non-retryable-data",
|
|
Usage: "Drop data when a non-retryable error is encountered instead of retrying",
|
|
Destination: ¶ms.DropNonRetryableData,
|
|
},
|
|
&cli.BoolFlag{
|
|
Name: "no-drop-non-retryable-data",
|
|
Usage: "Do not drop data when a non-retryable error is encountered",
|
|
Destination: ¶ms.NoDropNonRetryableData,
|
|
},
|
|
&cli.Int64Flag{
|
|
Name: "max-age",
|
|
Usage: "Specify a maximum age (in seconds) for replications data before it is dropped, or 0 for infinite",
|
|
Destination: ¶ms.MaxAge,
|
|
},
|
|
),
|
|
Action: func(ctx *cli.Context) error {
|
|
api := getAPI(ctx)
|
|
|
|
client := replication.Client{
|
|
CLI: getCLI(ctx),
|
|
ReplicationsApi: api.ReplicationsApi,
|
|
}
|
|
|
|
return client.Update(getContext(ctx), ¶ms)
|
|
},
|
|
}
|
|
}
|