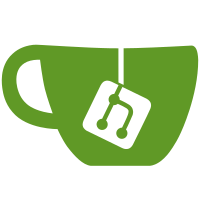
* basic list, create, and invoke working * all commands working * added support for create script body from file and invoke params from file * linter cleanup * update defaults to existing parameters if not provided * updated generated mock files, added mock files for scripts, added basic script create test * added mock script list * cleanup pass, fixed not using params in list call * added update mock test * fixed mock tests requiring go 1.18 * updated openapi, integrated overrides upstream, added new override to fix codegen bug * added nil check * fixed routes
114 lines
3.2 KiB
Go
114 lines
3.2 KiB
Go
/*
|
|
* Subset of Influx API covered by Influx CLI
|
|
*
|
|
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
|
|
*
|
|
* API version: 2.0.0
|
|
*/
|
|
|
|
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
|
|
|
|
package api
|
|
|
|
import (
|
|
"encoding/json"
|
|
)
|
|
|
|
// ScriptInvocationParams struct for ScriptInvocationParams
|
|
type ScriptInvocationParams struct {
|
|
Params *map[string]interface{} `json:"params,omitempty" yaml:"params,omitempty"`
|
|
}
|
|
|
|
// NewScriptInvocationParams instantiates a new ScriptInvocationParams object
|
|
// This constructor will assign default values to properties that have it defined,
|
|
// and makes sure properties required by API are set, but the set of arguments
|
|
// will change when the set of required properties is changed
|
|
func NewScriptInvocationParams() *ScriptInvocationParams {
|
|
this := ScriptInvocationParams{}
|
|
return &this
|
|
}
|
|
|
|
// NewScriptInvocationParamsWithDefaults instantiates a new ScriptInvocationParams object
|
|
// This constructor will only assign default values to properties that have it defined,
|
|
// but it doesn't guarantee that properties required by API are set
|
|
func NewScriptInvocationParamsWithDefaults() *ScriptInvocationParams {
|
|
this := ScriptInvocationParams{}
|
|
return &this
|
|
}
|
|
|
|
// GetParams returns the Params field value if set, zero value otherwise.
|
|
func (o *ScriptInvocationParams) GetParams() map[string]interface{} {
|
|
if o == nil || o.Params == nil {
|
|
var ret map[string]interface{}
|
|
return ret
|
|
}
|
|
return *o.Params
|
|
}
|
|
|
|
// GetParamsOk returns a tuple with the Params field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ScriptInvocationParams) GetParamsOk() (*map[string]interface{}, bool) {
|
|
if o == nil || o.Params == nil {
|
|
return nil, false
|
|
}
|
|
return o.Params, true
|
|
}
|
|
|
|
// HasParams returns a boolean if a field has been set.
|
|
func (o *ScriptInvocationParams) HasParams() bool {
|
|
if o != nil && o.Params != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetParams gets a reference to the given map[string]interface{} and assigns it to the Params field.
|
|
func (o *ScriptInvocationParams) SetParams(v map[string]interface{}) {
|
|
o.Params = &v
|
|
}
|
|
|
|
func (o ScriptInvocationParams) MarshalJSON() ([]byte, error) {
|
|
toSerialize := map[string]interface{}{}
|
|
if o.Params != nil {
|
|
toSerialize["params"] = o.Params
|
|
}
|
|
return json.Marshal(toSerialize)
|
|
}
|
|
|
|
type NullableScriptInvocationParams struct {
|
|
value *ScriptInvocationParams
|
|
isSet bool
|
|
}
|
|
|
|
func (v NullableScriptInvocationParams) Get() *ScriptInvocationParams {
|
|
return v.value
|
|
}
|
|
|
|
func (v *NullableScriptInvocationParams) Set(val *ScriptInvocationParams) {
|
|
v.value = val
|
|
v.isSet = true
|
|
}
|
|
|
|
func (v NullableScriptInvocationParams) IsSet() bool {
|
|
return v.isSet
|
|
}
|
|
|
|
func (v *NullableScriptInvocationParams) Unset() {
|
|
v.value = nil
|
|
v.isSet = false
|
|
}
|
|
|
|
func NewNullableScriptInvocationParams(val *ScriptInvocationParams) *NullableScriptInvocationParams {
|
|
return &NullableScriptInvocationParams{value: val, isSet: true}
|
|
}
|
|
|
|
func (v NullableScriptInvocationParams) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(v.value)
|
|
}
|
|
|
|
func (v *NullableScriptInvocationParams) UnmarshalJSON(src []byte) error {
|
|
v.isSet = true
|
|
return json.Unmarshal(src, &v.value)
|
|
}
|