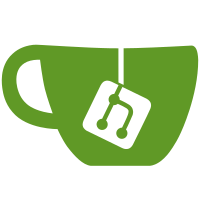
* refactor: take clients out of internal * refactor: move stdio to pkg * Move internal/api to api * refactor: final changes for Kapacitor to access shared functionality * chore: regenerate mocks * fix: bad automated refactor * chore: extra formatting not caught by make fmt
40 lines
1.0 KiB
Go
40 lines
1.0 KiB
Go
package api
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
// UnmarshalCSV implements the gocsv.TypeUnmarshaller interface
|
|
// for decoding CSV.
|
|
func (v *ColumnSemanticType) UnmarshalCSV(s string) error {
|
|
types := []string{string(COLUMNSEMANTICTYPE_TIMESTAMP), string(COLUMNSEMANTICTYPE_TAG), string(COLUMNSEMANTICTYPE_FIELD)}
|
|
for _, t := range types {
|
|
if s == t {
|
|
*v = ColumnSemanticType(t)
|
|
return nil
|
|
}
|
|
}
|
|
return fmt.Errorf("%q is not a valid column type. Valid values are [%s]", s, strings.Join(types, ", "))
|
|
}
|
|
|
|
// UnmarshalCSV implements the gocsv.TypeUnmarshaller interface
|
|
// for decoding CSV.
|
|
func (v *ColumnDataType) UnmarshalCSV(s string) error {
|
|
types := []string{
|
|
string(COLUMNDATATYPE_INTEGER),
|
|
string(COLUMNDATATYPE_FLOAT),
|
|
string(COLUMNDATATYPE_BOOLEAN),
|
|
string(COLUMNDATATYPE_STRING),
|
|
string(COLUMNDATATYPE_UNSIGNED),
|
|
}
|
|
|
|
for _, t := range types {
|
|
if s == t {
|
|
*v = ColumnDataType(t)
|
|
return nil
|
|
}
|
|
}
|
|
return fmt.Errorf("%q is not a valid column data type. Valid values are [%s]", s, strings.Join(types, ", "))
|
|
}
|