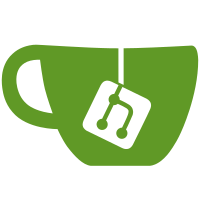
* add v1-compatible query path and refactor other paths to de-duplicate "/query" * add initial influxQL repl * add ping endpoint to schema * improve prompt UX, implement some commands * fix json column type in schema and improve completion * feat: add table formatter and move to forked go-prompt * improve formatting and add table pagination * implement more REPL commands, including insert and history * implement "INSERT INTO" * move repl command to "v1 repl" * refactor and improve documentation * clean up v1_repl cmd * update to latest openapi, use some openapi paths instead of overrides * remove additional files that were moved to openapi * compute historyFilePath at REPL start * clean up REPL use command logic flow * clean up comments for TODOs now in issues * move gopher (chonky boi) * remove autocompletion for separate PR * run go mod tidy * add rfc3339 precision option * allow left and right column scrolling to display whole table * add error to JSON query response * add tags and partial to JSON response series schema * fix csv formatting and add column formatting * remove table format for separate PR * fix getDatabases * move from write to legacy write endpoint for INSERT * remove history vestiges * allow multiple spaces in INSERT commands * add precision comment * remove auth for separate PR * separate parseInsert and add unit test * add additional test case and improve error messages * fix missing errors import * print rfc3339 precision * add rfc3339 to help output
999 lines
35 KiB
Go
999 lines
35 KiB
Go
/*
|
|
* Subset of Influx API covered by Influx CLI
|
|
*
|
|
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
|
|
*
|
|
* API version: 2.0.0
|
|
*/
|
|
|
|
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
|
|
|
|
package api
|
|
|
|
import (
|
|
_context "context"
|
|
_fmt "fmt"
|
|
_io "io"
|
|
_nethttp "net/http"
|
|
_neturl "net/url"
|
|
"strings"
|
|
)
|
|
|
|
// Linger please
|
|
var (
|
|
_ _context.Context
|
|
)
|
|
|
|
type AuthorizationsApi interface {
|
|
|
|
/*
|
|
* DeleteAuthorizationsID Delete an authorization
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param authID The ID of the authorization to delete.
|
|
* @return ApiDeleteAuthorizationsIDRequest
|
|
*/
|
|
DeleteAuthorizationsID(ctx _context.Context, authID string) ApiDeleteAuthorizationsIDRequest
|
|
|
|
/*
|
|
* DeleteAuthorizationsIDExecute executes the request
|
|
*/
|
|
DeleteAuthorizationsIDExecute(r ApiDeleteAuthorizationsIDRequest) error
|
|
|
|
/*
|
|
* DeleteAuthorizationsIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
*/
|
|
DeleteAuthorizationsIDExecuteWithHttpInfo(r ApiDeleteAuthorizationsIDRequest) (*_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetAuthorizations List all authorizations
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiGetAuthorizationsRequest
|
|
*/
|
|
GetAuthorizations(ctx _context.Context) ApiGetAuthorizationsRequest
|
|
|
|
/*
|
|
* GetAuthorizationsExecute executes the request
|
|
* @return Authorizations
|
|
*/
|
|
GetAuthorizationsExecute(r ApiGetAuthorizationsRequest) (Authorizations, error)
|
|
|
|
/*
|
|
* GetAuthorizationsExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Authorizations
|
|
*/
|
|
GetAuthorizationsExecuteWithHttpInfo(r ApiGetAuthorizationsRequest) (Authorizations, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetAuthorizationsID Retrieve an authorization
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param authID The ID of the authorization to get.
|
|
* @return ApiGetAuthorizationsIDRequest
|
|
*/
|
|
GetAuthorizationsID(ctx _context.Context, authID string) ApiGetAuthorizationsIDRequest
|
|
|
|
/*
|
|
* GetAuthorizationsIDExecute executes the request
|
|
* @return Authorization
|
|
*/
|
|
GetAuthorizationsIDExecute(r ApiGetAuthorizationsIDRequest) (Authorization, error)
|
|
|
|
/*
|
|
* GetAuthorizationsIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Authorization
|
|
*/
|
|
GetAuthorizationsIDExecuteWithHttpInfo(r ApiGetAuthorizationsIDRequest) (Authorization, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PatchAuthorizationsID Update an authorization to be active or inactive
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param authID The ID of the authorization to update.
|
|
* @return ApiPatchAuthorizationsIDRequest
|
|
*/
|
|
PatchAuthorizationsID(ctx _context.Context, authID string) ApiPatchAuthorizationsIDRequest
|
|
|
|
/*
|
|
* PatchAuthorizationsIDExecute executes the request
|
|
* @return Authorization
|
|
*/
|
|
PatchAuthorizationsIDExecute(r ApiPatchAuthorizationsIDRequest) (Authorization, error)
|
|
|
|
/*
|
|
* PatchAuthorizationsIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Authorization
|
|
*/
|
|
PatchAuthorizationsIDExecuteWithHttpInfo(r ApiPatchAuthorizationsIDRequest) (Authorization, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PostAuthorizations Create an authorization
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiPostAuthorizationsRequest
|
|
*/
|
|
PostAuthorizations(ctx _context.Context) ApiPostAuthorizationsRequest
|
|
|
|
/*
|
|
* PostAuthorizationsExecute executes the request
|
|
* @return Authorization
|
|
*/
|
|
PostAuthorizationsExecute(r ApiPostAuthorizationsRequest) (Authorization, error)
|
|
|
|
/*
|
|
* PostAuthorizationsExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return Authorization
|
|
*/
|
|
PostAuthorizationsExecuteWithHttpInfo(r ApiPostAuthorizationsRequest) (Authorization, *_nethttp.Response, error)
|
|
}
|
|
|
|
// AuthorizationsApiService AuthorizationsApi service
|
|
type AuthorizationsApiService service
|
|
|
|
type ApiDeleteAuthorizationsIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService AuthorizationsApi
|
|
authID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiDeleteAuthorizationsIDRequest) AuthID(authID string) ApiDeleteAuthorizationsIDRequest {
|
|
r.authID = authID
|
|
return r
|
|
}
|
|
func (r ApiDeleteAuthorizationsIDRequest) GetAuthID() string {
|
|
return r.authID
|
|
}
|
|
|
|
func (r ApiDeleteAuthorizationsIDRequest) ZapTraceSpan(zapTraceSpan string) ApiDeleteAuthorizationsIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiDeleteAuthorizationsIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiDeleteAuthorizationsIDRequest) Execute() error {
|
|
return r.ApiService.DeleteAuthorizationsIDExecute(r)
|
|
}
|
|
|
|
func (r ApiDeleteAuthorizationsIDRequest) ExecuteWithHttpInfo() (*_nethttp.Response, error) {
|
|
return r.ApiService.DeleteAuthorizationsIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* DeleteAuthorizationsID Delete an authorization
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param authID The ID of the authorization to delete.
|
|
* @return ApiDeleteAuthorizationsIDRequest
|
|
*/
|
|
func (a *AuthorizationsApiService) DeleteAuthorizationsID(ctx _context.Context, authID string) ApiDeleteAuthorizationsIDRequest {
|
|
return ApiDeleteAuthorizationsIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
authID: authID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
*/
|
|
func (a *AuthorizationsApiService) DeleteAuthorizationsIDExecute(r ApiDeleteAuthorizationsIDRequest) error {
|
|
_, err := a.DeleteAuthorizationsIDExecuteWithHttpInfo(r)
|
|
return err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
*/
|
|
func (a *AuthorizationsApiService) DeleteAuthorizationsIDExecuteWithHttpInfo(r ApiDeleteAuthorizationsIDRequest) (*_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodDelete
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "AuthorizationsApiService.DeleteAuthorizationsID")
|
|
if err != nil {
|
|
return nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/authorizations/{authID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"authID"+"}", _neturl.PathEscape(parameterToString(r.authID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetAuthorizationsRequest struct {
|
|
ctx _context.Context
|
|
ApiService AuthorizationsApi
|
|
zapTraceSpan *string
|
|
userID *string
|
|
user *string
|
|
orgID *string
|
|
org *string
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsRequest) ZapTraceSpan(zapTraceSpan string) ApiGetAuthorizationsRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetAuthorizationsRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsRequest) UserID(userID string) ApiGetAuthorizationsRequest {
|
|
r.userID = &userID
|
|
return r
|
|
}
|
|
func (r ApiGetAuthorizationsRequest) GetUserID() *string {
|
|
return r.userID
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsRequest) User(user string) ApiGetAuthorizationsRequest {
|
|
r.user = &user
|
|
return r
|
|
}
|
|
func (r ApiGetAuthorizationsRequest) GetUser() *string {
|
|
return r.user
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsRequest) OrgID(orgID string) ApiGetAuthorizationsRequest {
|
|
r.orgID = &orgID
|
|
return r
|
|
}
|
|
func (r ApiGetAuthorizationsRequest) GetOrgID() *string {
|
|
return r.orgID
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsRequest) Org(org string) ApiGetAuthorizationsRequest {
|
|
r.org = &org
|
|
return r
|
|
}
|
|
func (r ApiGetAuthorizationsRequest) GetOrg() *string {
|
|
return r.org
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsRequest) Execute() (Authorizations, error) {
|
|
return r.ApiService.GetAuthorizationsExecute(r)
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsRequest) ExecuteWithHttpInfo() (Authorizations, *_nethttp.Response, error) {
|
|
return r.ApiService.GetAuthorizationsExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* GetAuthorizations List all authorizations
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiGetAuthorizationsRequest
|
|
*/
|
|
func (a *AuthorizationsApiService) GetAuthorizations(ctx _context.Context) ApiGetAuthorizationsRequest {
|
|
return ApiGetAuthorizationsRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Authorizations
|
|
*/
|
|
func (a *AuthorizationsApiService) GetAuthorizationsExecute(r ApiGetAuthorizationsRequest) (Authorizations, error) {
|
|
returnVal, _, err := a.GetAuthorizationsExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Authorizations
|
|
*/
|
|
func (a *AuthorizationsApiService) GetAuthorizationsExecuteWithHttpInfo(r ApiGetAuthorizationsRequest) (Authorizations, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Authorizations
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "AuthorizationsApiService.GetAuthorizations")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/authorizations"
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
if r.userID != nil {
|
|
localVarQueryParams.Add("userID", parameterToString(*r.userID, ""))
|
|
}
|
|
if r.user != nil {
|
|
localVarQueryParams.Add("user", parameterToString(*r.user, ""))
|
|
}
|
|
if r.orgID != nil {
|
|
localVarQueryParams.Add("orgID", parameterToString(*r.orgID, ""))
|
|
}
|
|
if r.org != nil {
|
|
localVarQueryParams.Add("org", parameterToString(*r.org, ""))
|
|
}
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetAuthorizationsIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService AuthorizationsApi
|
|
authID string
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsIDRequest) AuthID(authID string) ApiGetAuthorizationsIDRequest {
|
|
r.authID = authID
|
|
return r
|
|
}
|
|
func (r ApiGetAuthorizationsIDRequest) GetAuthID() string {
|
|
return r.authID
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsIDRequest) ZapTraceSpan(zapTraceSpan string) ApiGetAuthorizationsIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetAuthorizationsIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsIDRequest) Execute() (Authorization, error) {
|
|
return r.ApiService.GetAuthorizationsIDExecute(r)
|
|
}
|
|
|
|
func (r ApiGetAuthorizationsIDRequest) ExecuteWithHttpInfo() (Authorization, *_nethttp.Response, error) {
|
|
return r.ApiService.GetAuthorizationsIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* GetAuthorizationsID Retrieve an authorization
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param authID The ID of the authorization to get.
|
|
* @return ApiGetAuthorizationsIDRequest
|
|
*/
|
|
func (a *AuthorizationsApiService) GetAuthorizationsID(ctx _context.Context, authID string) ApiGetAuthorizationsIDRequest {
|
|
return ApiGetAuthorizationsIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
authID: authID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Authorization
|
|
*/
|
|
func (a *AuthorizationsApiService) GetAuthorizationsIDExecute(r ApiGetAuthorizationsIDRequest) (Authorization, error) {
|
|
returnVal, _, err := a.GetAuthorizationsIDExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Authorization
|
|
*/
|
|
func (a *AuthorizationsApiService) GetAuthorizationsIDExecuteWithHttpInfo(r ApiGetAuthorizationsIDRequest) (Authorization, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Authorization
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "AuthorizationsApiService.GetAuthorizationsID")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/authorizations/{authID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"authID"+"}", _neturl.PathEscape(parameterToString(r.authID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPatchAuthorizationsIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService AuthorizationsApi
|
|
authID string
|
|
authorizationUpdateRequest *AuthorizationUpdateRequest
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiPatchAuthorizationsIDRequest) AuthID(authID string) ApiPatchAuthorizationsIDRequest {
|
|
r.authID = authID
|
|
return r
|
|
}
|
|
func (r ApiPatchAuthorizationsIDRequest) GetAuthID() string {
|
|
return r.authID
|
|
}
|
|
|
|
func (r ApiPatchAuthorizationsIDRequest) AuthorizationUpdateRequest(authorizationUpdateRequest AuthorizationUpdateRequest) ApiPatchAuthorizationsIDRequest {
|
|
r.authorizationUpdateRequest = &authorizationUpdateRequest
|
|
return r
|
|
}
|
|
func (r ApiPatchAuthorizationsIDRequest) GetAuthorizationUpdateRequest() *AuthorizationUpdateRequest {
|
|
return r.authorizationUpdateRequest
|
|
}
|
|
|
|
func (r ApiPatchAuthorizationsIDRequest) ZapTraceSpan(zapTraceSpan string) ApiPatchAuthorizationsIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPatchAuthorizationsIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPatchAuthorizationsIDRequest) Execute() (Authorization, error) {
|
|
return r.ApiService.PatchAuthorizationsIDExecute(r)
|
|
}
|
|
|
|
func (r ApiPatchAuthorizationsIDRequest) ExecuteWithHttpInfo() (Authorization, *_nethttp.Response, error) {
|
|
return r.ApiService.PatchAuthorizationsIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* PatchAuthorizationsID Update an authorization to be active or inactive
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param authID The ID of the authorization to update.
|
|
* @return ApiPatchAuthorizationsIDRequest
|
|
*/
|
|
func (a *AuthorizationsApiService) PatchAuthorizationsID(ctx _context.Context, authID string) ApiPatchAuthorizationsIDRequest {
|
|
return ApiPatchAuthorizationsIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
authID: authID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Authorization
|
|
*/
|
|
func (a *AuthorizationsApiService) PatchAuthorizationsIDExecute(r ApiPatchAuthorizationsIDRequest) (Authorization, error) {
|
|
returnVal, _, err := a.PatchAuthorizationsIDExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Authorization
|
|
*/
|
|
func (a *AuthorizationsApiService) PatchAuthorizationsIDExecuteWithHttpInfo(r ApiPatchAuthorizationsIDRequest) (Authorization, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPatch
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Authorization
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "AuthorizationsApiService.PatchAuthorizationsID")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/authorizations/{authID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"authID"+"}", _neturl.PathEscape(parameterToString(r.authID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
if r.authorizationUpdateRequest == nil {
|
|
return localVarReturnValue, nil, reportError("authorizationUpdateRequest is required and must be specified")
|
|
}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.authorizationUpdateRequest
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPostAuthorizationsRequest struct {
|
|
ctx _context.Context
|
|
ApiService AuthorizationsApi
|
|
authorizationPostRequest *AuthorizationPostRequest
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiPostAuthorizationsRequest) AuthorizationPostRequest(authorizationPostRequest AuthorizationPostRequest) ApiPostAuthorizationsRequest {
|
|
r.authorizationPostRequest = &authorizationPostRequest
|
|
return r
|
|
}
|
|
func (r ApiPostAuthorizationsRequest) GetAuthorizationPostRequest() *AuthorizationPostRequest {
|
|
return r.authorizationPostRequest
|
|
}
|
|
|
|
func (r ApiPostAuthorizationsRequest) ZapTraceSpan(zapTraceSpan string) ApiPostAuthorizationsRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPostAuthorizationsRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPostAuthorizationsRequest) Execute() (Authorization, error) {
|
|
return r.ApiService.PostAuthorizationsExecute(r)
|
|
}
|
|
|
|
func (r ApiPostAuthorizationsRequest) ExecuteWithHttpInfo() (Authorization, *_nethttp.Response, error) {
|
|
return r.ApiService.PostAuthorizationsExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* PostAuthorizations Create an authorization
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiPostAuthorizationsRequest
|
|
*/
|
|
func (a *AuthorizationsApiService) PostAuthorizations(ctx _context.Context) ApiPostAuthorizationsRequest {
|
|
return ApiPostAuthorizationsRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return Authorization
|
|
*/
|
|
func (a *AuthorizationsApiService) PostAuthorizationsExecute(r ApiPostAuthorizationsRequest) (Authorization, error) {
|
|
returnVal, _, err := a.PostAuthorizationsExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return Authorization
|
|
*/
|
|
func (a *AuthorizationsApiService) PostAuthorizationsExecuteWithHttpInfo(r ApiPostAuthorizationsRequest) (Authorization, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPost
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue Authorization
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "AuthorizationsApiService.PostAuthorizations")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/authorizations"
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
if r.authorizationPostRequest == nil {
|
|
return localVarReturnValue, nil, reportError("authorizationPostRequest is required and must be specified")
|
|
}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.authorizationPostRequest
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|