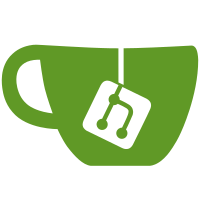
* add v1-compatible query path and refactor other paths to de-duplicate "/query" * add initial influxQL repl * add ping endpoint to schema * improve prompt UX, implement some commands * fix json column type in schema and improve completion * feat: add table formatter and move to forked go-prompt * improve formatting and add table pagination * implement more REPL commands, including insert and history * implement "INSERT INTO" * move repl command to "v1 repl" * refactor and improve documentation * clean up v1_repl cmd * update to latest openapi, use some openapi paths instead of overrides * remove additional files that were moved to openapi * compute historyFilePath at REPL start * clean up REPL use command logic flow * clean up comments for TODOs now in issues * move gopher (chonky boi) * remove autocompletion for separate PR * run go mod tidy * add rfc3339 precision option * allow left and right column scrolling to display whole table * add error to JSON query response * add tags and partial to JSON response series schema * fix csv formatting and add column formatting * remove table format for separate PR * fix getDatabases * move from write to legacy write endpoint for INSERT * remove history vestiges * allow multiple spaces in INSERT commands * add precision comment * remove auth for separate PR * separate parseInsert and add unit test * add additional test case and improve error messages * fix missing errors import * print rfc3339 precision * add rfc3339 to help output
1162 lines
37 KiB
Go
1162 lines
37 KiB
Go
/*
|
|
* Subset of Influx API covered by Influx CLI
|
|
*
|
|
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
|
|
*
|
|
* API version: 2.0.0
|
|
*/
|
|
|
|
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
|
|
|
|
package api
|
|
|
|
import (
|
|
_context "context"
|
|
_fmt "fmt"
|
|
_io "io"
|
|
_nethttp "net/http"
|
|
_neturl "net/url"
|
|
"strings"
|
|
)
|
|
|
|
// Linger please
|
|
var (
|
|
_ _context.Context
|
|
)
|
|
|
|
type DBRPsApi interface {
|
|
|
|
/*
|
|
* DeleteDBRPID Delete a database retention policy
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param dbrpID The database retention policy mapping
|
|
* @return ApiDeleteDBRPIDRequest
|
|
*/
|
|
DeleteDBRPID(ctx _context.Context, dbrpID string) ApiDeleteDBRPIDRequest
|
|
|
|
/*
|
|
* DeleteDBRPIDExecute executes the request
|
|
*/
|
|
DeleteDBRPIDExecute(r ApiDeleteDBRPIDRequest) error
|
|
|
|
/*
|
|
* DeleteDBRPIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
*/
|
|
DeleteDBRPIDExecuteWithHttpInfo(r ApiDeleteDBRPIDRequest) (*_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetDBRPs List database retention policy mappings
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiGetDBRPsRequest
|
|
*/
|
|
GetDBRPs(ctx _context.Context) ApiGetDBRPsRequest
|
|
|
|
/*
|
|
* GetDBRPsExecute executes the request
|
|
* @return DBRPs
|
|
*/
|
|
GetDBRPsExecute(r ApiGetDBRPsRequest) (DBRPs, error)
|
|
|
|
/*
|
|
* GetDBRPsExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return DBRPs
|
|
*/
|
|
GetDBRPsExecuteWithHttpInfo(r ApiGetDBRPsRequest) (DBRPs, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* GetDBRPsID Retrieve a database retention policy mapping
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param dbrpID The database retention policy mapping ID
|
|
* @return ApiGetDBRPsIDRequest
|
|
*/
|
|
GetDBRPsID(ctx _context.Context, dbrpID string) ApiGetDBRPsIDRequest
|
|
|
|
/*
|
|
* GetDBRPsIDExecute executes the request
|
|
* @return DBRPGet
|
|
*/
|
|
GetDBRPsIDExecute(r ApiGetDBRPsIDRequest) (DBRPGet, error)
|
|
|
|
/*
|
|
* GetDBRPsIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return DBRPGet
|
|
*/
|
|
GetDBRPsIDExecuteWithHttpInfo(r ApiGetDBRPsIDRequest) (DBRPGet, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PatchDBRPID Update a database retention policy mapping
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param dbrpID The database retention policy mapping.
|
|
* @return ApiPatchDBRPIDRequest
|
|
*/
|
|
PatchDBRPID(ctx _context.Context, dbrpID string) ApiPatchDBRPIDRequest
|
|
|
|
/*
|
|
* PatchDBRPIDExecute executes the request
|
|
* @return DBRPGet
|
|
*/
|
|
PatchDBRPIDExecute(r ApiPatchDBRPIDRequest) (DBRPGet, error)
|
|
|
|
/*
|
|
* PatchDBRPIDExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return DBRPGet
|
|
*/
|
|
PatchDBRPIDExecuteWithHttpInfo(r ApiPatchDBRPIDRequest) (DBRPGet, *_nethttp.Response, error)
|
|
|
|
/*
|
|
* PostDBRP Add a database retention policy mapping
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiPostDBRPRequest
|
|
*/
|
|
PostDBRP(ctx _context.Context) ApiPostDBRPRequest
|
|
|
|
/*
|
|
* PostDBRPExecute executes the request
|
|
* @return DBRP
|
|
*/
|
|
PostDBRPExecute(r ApiPostDBRPRequest) (DBRP, error)
|
|
|
|
/*
|
|
* PostDBRPExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not
|
|
* available on the returned HTTP response as it will have already been read and closed; access to the response body
|
|
* content should be achieved through the returned response model if applicable.
|
|
* @return DBRP
|
|
*/
|
|
PostDBRPExecuteWithHttpInfo(r ApiPostDBRPRequest) (DBRP, *_nethttp.Response, error)
|
|
}
|
|
|
|
// DBRPsApiService DBRPsApi service
|
|
type DBRPsApiService service
|
|
|
|
type ApiDeleteDBRPIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService DBRPsApi
|
|
dbrpID string
|
|
zapTraceSpan *string
|
|
orgID *string
|
|
org *string
|
|
}
|
|
|
|
func (r ApiDeleteDBRPIDRequest) DbrpID(dbrpID string) ApiDeleteDBRPIDRequest {
|
|
r.dbrpID = dbrpID
|
|
return r
|
|
}
|
|
func (r ApiDeleteDBRPIDRequest) GetDbrpID() string {
|
|
return r.dbrpID
|
|
}
|
|
|
|
func (r ApiDeleteDBRPIDRequest) ZapTraceSpan(zapTraceSpan string) ApiDeleteDBRPIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiDeleteDBRPIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiDeleteDBRPIDRequest) OrgID(orgID string) ApiDeleteDBRPIDRequest {
|
|
r.orgID = &orgID
|
|
return r
|
|
}
|
|
func (r ApiDeleteDBRPIDRequest) GetOrgID() *string {
|
|
return r.orgID
|
|
}
|
|
|
|
func (r ApiDeleteDBRPIDRequest) Org(org string) ApiDeleteDBRPIDRequest {
|
|
r.org = &org
|
|
return r
|
|
}
|
|
func (r ApiDeleteDBRPIDRequest) GetOrg() *string {
|
|
return r.org
|
|
}
|
|
|
|
func (r ApiDeleteDBRPIDRequest) Execute() error {
|
|
return r.ApiService.DeleteDBRPIDExecute(r)
|
|
}
|
|
|
|
func (r ApiDeleteDBRPIDRequest) ExecuteWithHttpInfo() (*_nethttp.Response, error) {
|
|
return r.ApiService.DeleteDBRPIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* DeleteDBRPID Delete a database retention policy
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param dbrpID The database retention policy mapping
|
|
* @return ApiDeleteDBRPIDRequest
|
|
*/
|
|
func (a *DBRPsApiService) DeleteDBRPID(ctx _context.Context, dbrpID string) ApiDeleteDBRPIDRequest {
|
|
return ApiDeleteDBRPIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
dbrpID: dbrpID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
*/
|
|
func (a *DBRPsApiService) DeleteDBRPIDExecute(r ApiDeleteDBRPIDRequest) error {
|
|
_, err := a.DeleteDBRPIDExecuteWithHttpInfo(r)
|
|
return err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
*/
|
|
func (a *DBRPsApiService) DeleteDBRPIDExecuteWithHttpInfo(r ApiDeleteDBRPIDRequest) (*_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodDelete
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "DBRPsApiService.DeleteDBRPID")
|
|
if err != nil {
|
|
return nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/dbrps/{dbrpID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"dbrpID"+"}", _neturl.PathEscape(parameterToString(r.dbrpID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
if r.orgID != nil {
|
|
localVarQueryParams.Add("orgID", parameterToString(*r.orgID, ""))
|
|
}
|
|
if r.org != nil {
|
|
localVarQueryParams.Add("org", parameterToString(*r.org, ""))
|
|
}
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetDBRPsRequest struct {
|
|
ctx _context.Context
|
|
ApiService DBRPsApi
|
|
zapTraceSpan *string
|
|
orgID *string
|
|
org *string
|
|
id *string
|
|
bucketID *string
|
|
default_ *bool
|
|
db *string
|
|
rp *string
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) ZapTraceSpan(zapTraceSpan string) ApiGetDBRPsRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) OrgID(orgID string) ApiGetDBRPsRequest {
|
|
r.orgID = &orgID
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetOrgID() *string {
|
|
return r.orgID
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) Org(org string) ApiGetDBRPsRequest {
|
|
r.org = &org
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetOrg() *string {
|
|
return r.org
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) Id(id string) ApiGetDBRPsRequest {
|
|
r.id = &id
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetId() *string {
|
|
return r.id
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) BucketID(bucketID string) ApiGetDBRPsRequest {
|
|
r.bucketID = &bucketID
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetBucketID() *string {
|
|
return r.bucketID
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) Default_(default_ bool) ApiGetDBRPsRequest {
|
|
r.default_ = &default_
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetDefault_() *bool {
|
|
return r.default_
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) Db(db string) ApiGetDBRPsRequest {
|
|
r.db = &db
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetDb() *string {
|
|
return r.db
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) Rp(rp string) ApiGetDBRPsRequest {
|
|
r.rp = &rp
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsRequest) GetRp() *string {
|
|
return r.rp
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) Execute() (DBRPs, error) {
|
|
return r.ApiService.GetDBRPsExecute(r)
|
|
}
|
|
|
|
func (r ApiGetDBRPsRequest) ExecuteWithHttpInfo() (DBRPs, *_nethttp.Response, error) {
|
|
return r.ApiService.GetDBRPsExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* GetDBRPs List database retention policy mappings
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiGetDBRPsRequest
|
|
*/
|
|
func (a *DBRPsApiService) GetDBRPs(ctx _context.Context) ApiGetDBRPsRequest {
|
|
return ApiGetDBRPsRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return DBRPs
|
|
*/
|
|
func (a *DBRPsApiService) GetDBRPsExecute(r ApiGetDBRPsRequest) (DBRPs, error) {
|
|
returnVal, _, err := a.GetDBRPsExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return DBRPs
|
|
*/
|
|
func (a *DBRPsApiService) GetDBRPsExecuteWithHttpInfo(r ApiGetDBRPsRequest) (DBRPs, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue DBRPs
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "DBRPsApiService.GetDBRPs")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/dbrps"
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
if r.orgID != nil {
|
|
localVarQueryParams.Add("orgID", parameterToString(*r.orgID, ""))
|
|
}
|
|
if r.org != nil {
|
|
localVarQueryParams.Add("org", parameterToString(*r.org, ""))
|
|
}
|
|
if r.id != nil {
|
|
localVarQueryParams.Add("id", parameterToString(*r.id, ""))
|
|
}
|
|
if r.bucketID != nil {
|
|
localVarQueryParams.Add("bucketID", parameterToString(*r.bucketID, ""))
|
|
}
|
|
if r.default_ != nil {
|
|
localVarQueryParams.Add("default", parameterToString(*r.default_, ""))
|
|
}
|
|
if r.db != nil {
|
|
localVarQueryParams.Add("db", parameterToString(*r.db, ""))
|
|
}
|
|
if r.rp != nil {
|
|
localVarQueryParams.Add("rp", parameterToString(*r.rp, ""))
|
|
}
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiGetDBRPsIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService DBRPsApi
|
|
dbrpID string
|
|
zapTraceSpan *string
|
|
orgID *string
|
|
org *string
|
|
}
|
|
|
|
func (r ApiGetDBRPsIDRequest) DbrpID(dbrpID string) ApiGetDBRPsIDRequest {
|
|
r.dbrpID = dbrpID
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsIDRequest) GetDbrpID() string {
|
|
return r.dbrpID
|
|
}
|
|
|
|
func (r ApiGetDBRPsIDRequest) ZapTraceSpan(zapTraceSpan string) ApiGetDBRPsIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiGetDBRPsIDRequest) OrgID(orgID string) ApiGetDBRPsIDRequest {
|
|
r.orgID = &orgID
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsIDRequest) GetOrgID() *string {
|
|
return r.orgID
|
|
}
|
|
|
|
func (r ApiGetDBRPsIDRequest) Org(org string) ApiGetDBRPsIDRequest {
|
|
r.org = &org
|
|
return r
|
|
}
|
|
func (r ApiGetDBRPsIDRequest) GetOrg() *string {
|
|
return r.org
|
|
}
|
|
|
|
func (r ApiGetDBRPsIDRequest) Execute() (DBRPGet, error) {
|
|
return r.ApiService.GetDBRPsIDExecute(r)
|
|
}
|
|
|
|
func (r ApiGetDBRPsIDRequest) ExecuteWithHttpInfo() (DBRPGet, *_nethttp.Response, error) {
|
|
return r.ApiService.GetDBRPsIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* GetDBRPsID Retrieve a database retention policy mapping
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param dbrpID The database retention policy mapping ID
|
|
* @return ApiGetDBRPsIDRequest
|
|
*/
|
|
func (a *DBRPsApiService) GetDBRPsID(ctx _context.Context, dbrpID string) ApiGetDBRPsIDRequest {
|
|
return ApiGetDBRPsIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
dbrpID: dbrpID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return DBRPGet
|
|
*/
|
|
func (a *DBRPsApiService) GetDBRPsIDExecute(r ApiGetDBRPsIDRequest) (DBRPGet, error) {
|
|
returnVal, _, err := a.GetDBRPsIDExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return DBRPGet
|
|
*/
|
|
func (a *DBRPsApiService) GetDBRPsIDExecuteWithHttpInfo(r ApiGetDBRPsIDRequest) (DBRPGet, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodGet
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue DBRPGet
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "DBRPsApiService.GetDBRPsID")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/dbrps/{dbrpID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"dbrpID"+"}", _neturl.PathEscape(parameterToString(r.dbrpID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
|
|
if r.orgID != nil {
|
|
localVarQueryParams.Add("orgID", parameterToString(*r.orgID, ""))
|
|
}
|
|
if r.org != nil {
|
|
localVarQueryParams.Add("org", parameterToString(*r.org, ""))
|
|
}
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPatchDBRPIDRequest struct {
|
|
ctx _context.Context
|
|
ApiService DBRPsApi
|
|
dbrpID string
|
|
dBRPUpdate *DBRPUpdate
|
|
zapTraceSpan *string
|
|
orgID *string
|
|
org *string
|
|
}
|
|
|
|
func (r ApiPatchDBRPIDRequest) DbrpID(dbrpID string) ApiPatchDBRPIDRequest {
|
|
r.dbrpID = dbrpID
|
|
return r
|
|
}
|
|
func (r ApiPatchDBRPIDRequest) GetDbrpID() string {
|
|
return r.dbrpID
|
|
}
|
|
|
|
func (r ApiPatchDBRPIDRequest) DBRPUpdate(dBRPUpdate DBRPUpdate) ApiPatchDBRPIDRequest {
|
|
r.dBRPUpdate = &dBRPUpdate
|
|
return r
|
|
}
|
|
func (r ApiPatchDBRPIDRequest) GetDBRPUpdate() *DBRPUpdate {
|
|
return r.dBRPUpdate
|
|
}
|
|
|
|
func (r ApiPatchDBRPIDRequest) ZapTraceSpan(zapTraceSpan string) ApiPatchDBRPIDRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPatchDBRPIDRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPatchDBRPIDRequest) OrgID(orgID string) ApiPatchDBRPIDRequest {
|
|
r.orgID = &orgID
|
|
return r
|
|
}
|
|
func (r ApiPatchDBRPIDRequest) GetOrgID() *string {
|
|
return r.orgID
|
|
}
|
|
|
|
func (r ApiPatchDBRPIDRequest) Org(org string) ApiPatchDBRPIDRequest {
|
|
r.org = &org
|
|
return r
|
|
}
|
|
func (r ApiPatchDBRPIDRequest) GetOrg() *string {
|
|
return r.org
|
|
}
|
|
|
|
func (r ApiPatchDBRPIDRequest) Execute() (DBRPGet, error) {
|
|
return r.ApiService.PatchDBRPIDExecute(r)
|
|
}
|
|
|
|
func (r ApiPatchDBRPIDRequest) ExecuteWithHttpInfo() (DBRPGet, *_nethttp.Response, error) {
|
|
return r.ApiService.PatchDBRPIDExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* PatchDBRPID Update a database retention policy mapping
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @param dbrpID The database retention policy mapping.
|
|
* @return ApiPatchDBRPIDRequest
|
|
*/
|
|
func (a *DBRPsApiService) PatchDBRPID(ctx _context.Context, dbrpID string) ApiPatchDBRPIDRequest {
|
|
return ApiPatchDBRPIDRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
dbrpID: dbrpID,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return DBRPGet
|
|
*/
|
|
func (a *DBRPsApiService) PatchDBRPIDExecute(r ApiPatchDBRPIDRequest) (DBRPGet, error) {
|
|
returnVal, _, err := a.PatchDBRPIDExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return DBRPGet
|
|
*/
|
|
func (a *DBRPsApiService) PatchDBRPIDExecuteWithHttpInfo(r ApiPatchDBRPIDRequest) (DBRPGet, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPatch
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue DBRPGet
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "DBRPsApiService.PatchDBRPID")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/dbrps/{dbrpID}"
|
|
localVarPath = strings.Replace(localVarPath, "{"+"dbrpID"+"}", _neturl.PathEscape(parameterToString(r.dbrpID, "")), -1)
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
if r.dBRPUpdate == nil {
|
|
return localVarReturnValue, nil, reportError("dBRPUpdate is required and must be specified")
|
|
}
|
|
|
|
if r.orgID != nil {
|
|
localVarQueryParams.Add("orgID", parameterToString(*r.orgID, ""))
|
|
}
|
|
if r.org != nil {
|
|
localVarQueryParams.Add("org", parameterToString(*r.org, ""))
|
|
}
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.dBRPUpdate
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
if localVarHTTPResponse.StatusCode == 404 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|
|
|
|
type ApiPostDBRPRequest struct {
|
|
ctx _context.Context
|
|
ApiService DBRPsApi
|
|
dBRPCreate *DBRPCreate
|
|
zapTraceSpan *string
|
|
}
|
|
|
|
func (r ApiPostDBRPRequest) DBRPCreate(dBRPCreate DBRPCreate) ApiPostDBRPRequest {
|
|
r.dBRPCreate = &dBRPCreate
|
|
return r
|
|
}
|
|
func (r ApiPostDBRPRequest) GetDBRPCreate() *DBRPCreate {
|
|
return r.dBRPCreate
|
|
}
|
|
|
|
func (r ApiPostDBRPRequest) ZapTraceSpan(zapTraceSpan string) ApiPostDBRPRequest {
|
|
r.zapTraceSpan = &zapTraceSpan
|
|
return r
|
|
}
|
|
func (r ApiPostDBRPRequest) GetZapTraceSpan() *string {
|
|
return r.zapTraceSpan
|
|
}
|
|
|
|
func (r ApiPostDBRPRequest) Execute() (DBRP, error) {
|
|
return r.ApiService.PostDBRPExecute(r)
|
|
}
|
|
|
|
func (r ApiPostDBRPRequest) ExecuteWithHttpInfo() (DBRP, *_nethttp.Response, error) {
|
|
return r.ApiService.PostDBRPExecuteWithHttpInfo(r)
|
|
}
|
|
|
|
/*
|
|
* PostDBRP Add a database retention policy mapping
|
|
* @param ctx _context.Context - for authentication, logging, cancellation, deadlines, tracing, etc. Passed from http.Request or context.Background().
|
|
* @return ApiPostDBRPRequest
|
|
*/
|
|
func (a *DBRPsApiService) PostDBRP(ctx _context.Context) ApiPostDBRPRequest {
|
|
return ApiPostDBRPRequest{
|
|
ApiService: a,
|
|
ctx: ctx,
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Execute executes the request
|
|
* @return DBRP
|
|
*/
|
|
func (a *DBRPsApiService) PostDBRPExecute(r ApiPostDBRPRequest) (DBRP, error) {
|
|
returnVal, _, err := a.PostDBRPExecuteWithHttpInfo(r)
|
|
return returnVal, err
|
|
}
|
|
|
|
/*
|
|
* ExecuteWithHttpInfo executes the request with HTTP response info returned. The response body is not available on the
|
|
* returned HTTP response as it will have already been read and closed; access to the response body content should be
|
|
* achieved through the returned response model if applicable.
|
|
* @return DBRP
|
|
*/
|
|
func (a *DBRPsApiService) PostDBRPExecuteWithHttpInfo(r ApiPostDBRPRequest) (DBRP, *_nethttp.Response, error) {
|
|
var (
|
|
localVarHTTPMethod = _nethttp.MethodPost
|
|
localVarPostBody interface{}
|
|
localVarFormFileName string
|
|
localVarFileName string
|
|
localVarFileBytes []byte
|
|
localVarReturnValue DBRP
|
|
)
|
|
|
|
localBasePath, err := a.client.cfg.ServerURLWithContext(r.ctx, "DBRPsApiService.PostDBRP")
|
|
if err != nil {
|
|
return localVarReturnValue, nil, GenericOpenAPIError{error: err.Error()}
|
|
}
|
|
|
|
localVarPath := localBasePath + "/api/v2/dbrps"
|
|
|
|
localVarHeaderParams := make(map[string]string)
|
|
localVarQueryParams := _neturl.Values{}
|
|
localVarFormParams := _neturl.Values{}
|
|
if r.dBRPCreate == nil {
|
|
return localVarReturnValue, nil, reportError("dBRPCreate is required and must be specified")
|
|
}
|
|
|
|
// to determine the Content-Type header
|
|
localVarHTTPContentTypes := []string{"application/json"}
|
|
|
|
// set Content-Type header
|
|
localVarHTTPContentType := selectHeaderContentType(localVarHTTPContentTypes)
|
|
if localVarHTTPContentType != "" {
|
|
localVarHeaderParams["Content-Type"] = localVarHTTPContentType
|
|
}
|
|
|
|
// to determine the Accept header
|
|
localVarHTTPHeaderAccepts := []string{"application/json"}
|
|
|
|
// set Accept header
|
|
localVarHTTPHeaderAccept := selectHeaderAccept(localVarHTTPHeaderAccepts)
|
|
if localVarHTTPHeaderAccept != "" {
|
|
localVarHeaderParams["Accept"] = localVarHTTPHeaderAccept
|
|
}
|
|
if r.zapTraceSpan != nil {
|
|
localVarHeaderParams["Zap-Trace-Span"] = parameterToString(*r.zapTraceSpan, "")
|
|
}
|
|
// body params
|
|
localVarPostBody = r.dBRPCreate
|
|
req, err := a.client.prepareRequest(r.ctx, localVarPath, localVarHTTPMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFormFileName, localVarFileName, localVarFileBytes)
|
|
if err != nil {
|
|
return localVarReturnValue, nil, err
|
|
}
|
|
|
|
localVarHTTPResponse, err := a.client.callAPI(req)
|
|
if err != nil || localVarHTTPResponse == nil {
|
|
return localVarReturnValue, localVarHTTPResponse, err
|
|
}
|
|
|
|
newErr := GenericOpenAPIError{
|
|
buildHeader: localVarHTTPResponse.Header.Get("X-Influxdb-Build"),
|
|
}
|
|
|
|
if localVarHTTPResponse.StatusCode >= 300 {
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
newErr.error = localVarHTTPResponse.Status
|
|
if localVarHTTPResponse.StatusCode == 400 {
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
var v Error
|
|
err = a.client.decode(&v, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = _fmt.Sprintf("%s: %s", newErr.Error(), err.Error())
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
v.SetMessage(_fmt.Sprintf("%s: %s", newErr.Error(), v.GetMessage()))
|
|
newErr.model = &v
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
body, err := GunzipIfNeeded(localVarHTTPResponse)
|
|
if err != nil {
|
|
body.Close()
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
localVarBody, err := _io.ReadAll(body)
|
|
body.Close()
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
newErr.body = localVarBody
|
|
err = a.client.decode(&localVarReturnValue, localVarBody, localVarHTTPResponse.Header.Get("Content-Type"))
|
|
if err != nil {
|
|
newErr.error = err.Error()
|
|
return localVarReturnValue, localVarHTTPResponse, newErr
|
|
}
|
|
|
|
return localVarReturnValue, localVarHTTPResponse, nil
|
|
}
|