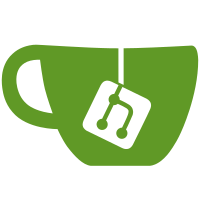
Influxdata cloud2 uses a different mechanism to enable tracing. You need to pass a `influx-debug-id` header (the value is ignored) and if the response contains `trace-sampled: true` then the response's `trace-id` header contains a valid trace ID that can be used to jump to the trace in jaeger. If the request has been sampled, we print the trace-id to stderr. Since modern terminals allow to click on hyperlinks, we could avoid a copy&paste of the trace id if the `influx` binary wrote a the link to the jeager trace. However, we don't want to hardcode the URL of the internal jaeger endpoint in the binary. I tried adding a wrapper script on my machine that would just capture the trace-id and render it, but it's hard to do without messing with the ordering of the stderr and stdout lines. Thus I preferred adding a secret env var that controls the formatting of the trace-id and can be used by our devs to generate links to our internal jaeger instance. ```console $ export INFLUX_CLI_TRACE_PRINT_PREFIX="Trace: https://jaeger.my.cloud/trace/" $ influx query --trace-debug-id 123 -f query.flux Trace: https://jaeger.my.cloud/trace/f39a69354a3acca6 Result: _result Table: keys: [_measurement] ... ```
46 lines
1.5 KiB
Go
46 lines
1.5 KiB
Go
package api
|
|
|
|
import (
|
|
"crypto/tls"
|
|
"fmt"
|
|
"net/http"
|
|
"net/url"
|
|
)
|
|
|
|
type ConfigParams struct {
|
|
Host *url.URL
|
|
UserAgent string
|
|
Token *string
|
|
TraceId *string
|
|
AllowInsecureTLS bool
|
|
Debug bool
|
|
}
|
|
|
|
// NewAPIConfig builds a configuration tailored to the InfluxDB v2 API.
|
|
func NewAPIConfig(params ConfigParams) *Configuration {
|
|
clientTransport := http.DefaultTransport.(*http.Transport).Clone()
|
|
clientTransport.TLSClientConfig = &tls.Config{InsecureSkipVerify: params.AllowInsecureTLS}
|
|
|
|
apiConfig := NewConfiguration()
|
|
apiConfig.Host = params.Host.Host
|
|
apiConfig.Scheme = params.Host.Scheme
|
|
apiConfig.UserAgent = params.UserAgent
|
|
apiConfig.HTTPClient = &http.Client{Transport: clientTransport}
|
|
if params.Token != nil {
|
|
apiConfig.DefaultHeader["Authorization"] = fmt.Sprintf("Token %s", *params.Token)
|
|
}
|
|
if params.TraceId != nil {
|
|
// NOTE: This is circumventing our codegen. If the header we use for tracing ever changes,
|
|
// we'll need to manually update the string here to match.
|
|
//
|
|
// The alternative is to pass the trace ID to the business logic for every CLI command, and
|
|
// use codegen'd logic to set the header on every HTTP request. Early versions of the CLI
|
|
// used that technique, and we found it to be error-prone and easy to forget during testing.
|
|
apiConfig.DefaultHeader["Zap-Trace-Span"] = *params.TraceId
|
|
apiConfig.DefaultHeader["influx-debug-id"] = *params.TraceId
|
|
}
|
|
apiConfig.Debug = params.Debug
|
|
|
|
return apiConfig
|
|
}
|