MXS-1220: Add server and session printing to REST API
The REST API now prints individual sessions and servers. It also lists all servers if no specific server is given. The functions directly call the printing functions when they should be using the inter-thread messaging system. When the messaging system is ready, these functions should be updated.
This commit is contained in:
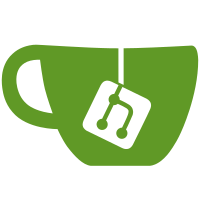
committed by
Markus Mäkelä
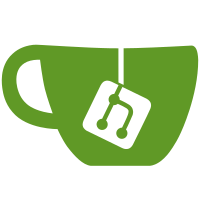
parent
fd680544d6
commit
9468893048
@ -13,8 +13,12 @@
|
||||
*/
|
||||
|
||||
#include <maxscale/cppdefs.hh>
|
||||
|
||||
#include <string>
|
||||
|
||||
#include <maxscale/jansson.h>
|
||||
#include <maxscale/utils.hh>
|
||||
#include <maxscale/alloc.h>
|
||||
|
||||
namespace maxscale
|
||||
{
|
||||
@ -41,4 +45,30 @@ struct CloserTraits<json_t*>
|
||||
}
|
||||
};
|
||||
|
||||
/**
|
||||
* @brief Convenience function for dumping JSON into a string
|
||||
*
|
||||
* @param json JSON to dump
|
||||
*
|
||||
* @return The JSON in string format
|
||||
*/
|
||||
static inline std::string json_dump(const json_t* json, int flags = 0)
|
||||
{
|
||||
std::string rval;
|
||||
char* js = json_dumps(json, flags);
|
||||
|
||||
if (js)
|
||||
{
|
||||
rval = js;
|
||||
MXS_FREE(js);
|
||||
}
|
||||
|
||||
return rval;
|
||||
}
|
||||
|
||||
static inline std::string json_dump(const Closer<json_t*>& json, int flags = 0)
|
||||
{
|
||||
return json_dump(json.get(), flags);
|
||||
}
|
||||
|
||||
}
|
||||
|
@ -13,6 +13,9 @@
|
||||
|
||||
#include <maxscale/spinlock.hh>
|
||||
|
||||
#include <maxscale/alloc.h>
|
||||
#include <maxscale/jansson.hh>
|
||||
|
||||
#include "maxscale/resource.hh"
|
||||
#include "maxscale/httprequest.hh"
|
||||
#include "maxscale/httpresponse.hh"
|
||||
@ -41,10 +44,13 @@ class ServersResource: public Resource
|
||||
protected:
|
||||
HttpResponse handle(HttpRequest& request)
|
||||
{
|
||||
int flags = request.get_option("pretty") == "true" ? JSON_INDENT(4) : 0;
|
||||
|
||||
if (request.uri_part_count() == 1)
|
||||
{
|
||||
// Show all servers
|
||||
return HttpResponse(HTTP_200_OK);
|
||||
// TODO: Generate this via the inter-thread messaging system
|
||||
Closer<json_t*> servers(server_list_to_json());
|
||||
return HttpResponse(HTTP_200_OK, mxs::json_dump(servers, flags));
|
||||
}
|
||||
else
|
||||
{
|
||||
@ -52,8 +58,10 @@ protected:
|
||||
|
||||
if (server)
|
||||
{
|
||||
// TODO: Generate this via the inter-thread messaging system
|
||||
Closer<json_t*> server_js(server_to_json(server));
|
||||
// Show one server
|
||||
return HttpResponse(HTTP_200_OK);
|
||||
return HttpResponse(HTTP_200_OK, mxs::json_dump(server_js, flags));
|
||||
}
|
||||
else
|
||||
{
|
||||
@ -161,9 +169,12 @@ protected:
|
||||
|
||||
if (session)
|
||||
{
|
||||
int flags = request.get_option("pretty") == "true" ? JSON_INDENT(4) : 0;
|
||||
// TODO: Generate this via the inter-thread messaging system
|
||||
Closer<json_t*> ses_json(session_to_json(session));
|
||||
session_put_ref(session);
|
||||
// Show session statistics
|
||||
return HttpResponse(HTTP_200_OK);
|
||||
return HttpResponse(HTTP_200_OK, mxs::json_dump(ses_json, flags));
|
||||
}
|
||||
else
|
||||
{
|
||||
|
Reference in New Issue
Block a user